mirror of
https://github.com/Unleash/unleash.git
synced 2025-05-17 01:17:29 +02:00
feat: export events as json (#7841)
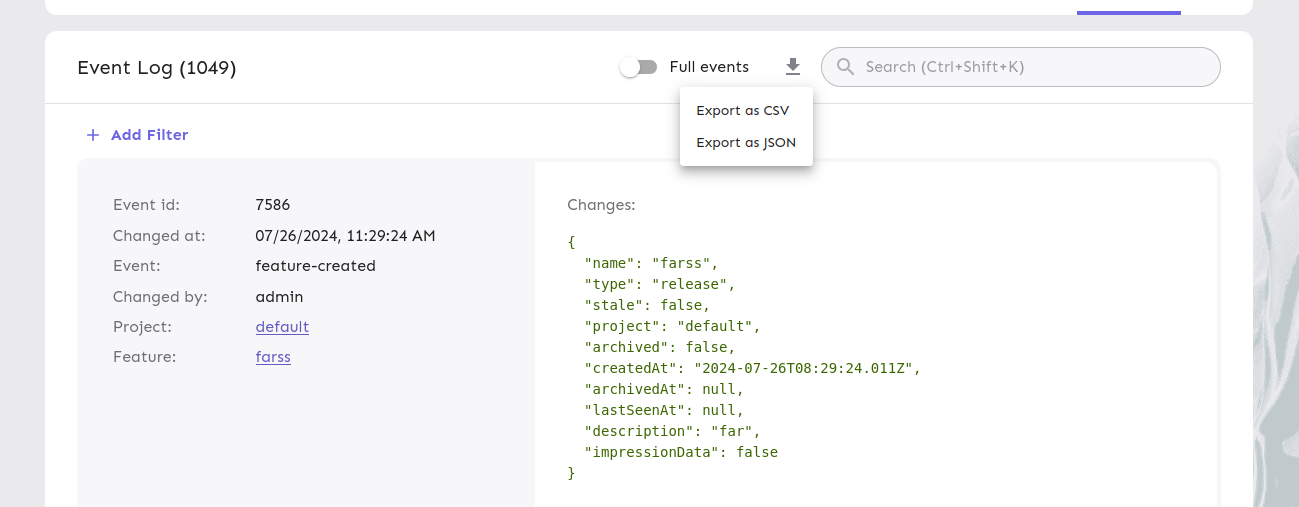
This commit is contained in:
parent
fa96ff1450
commit
b194393dae
108
frontend/src/component/events/EventLog/EventActions.tsx
Normal file
108
frontend/src/component/events/EventLog/EventActions.tsx
Normal file
@ -0,0 +1,108 @@
|
||||
import { type FC, useState } from 'react';
|
||||
import {
|
||||
IconButton,
|
||||
ListItemText,
|
||||
MenuItem,
|
||||
MenuList,
|
||||
Popover,
|
||||
styled,
|
||||
Tooltip,
|
||||
Typography,
|
||||
} from '@mui/material';
|
||||
import FileDownload from '@mui/icons-material/FileDownload';
|
||||
import type { EventSchema } from '../../../openapi';
|
||||
|
||||
const StyledActions = styled('div')(({ theme }) => ({
|
||||
display: 'flex',
|
||||
justifyContent: 'center',
|
||||
}));
|
||||
|
||||
const StyledPopover = styled(Popover)(({ theme }) => ({
|
||||
borderRadius: theme.shape.borderRadiusLarge,
|
||||
padding: theme.spacing(1, 1.5),
|
||||
}));
|
||||
|
||||
interface IEventActions {
|
||||
events: EventSchema[];
|
||||
}
|
||||
|
||||
export const EventActions: FC<IEventActions> = ({ events }) => {
|
||||
const [anchorEl, setAnchorEl] = useState<null | HTMLElement>(null);
|
||||
|
||||
const open = Boolean(anchorEl);
|
||||
const handleClick = (event: React.MouseEvent<HTMLButtonElement>) => {
|
||||
setAnchorEl(event.currentTarget);
|
||||
};
|
||||
const handleClose = () => {
|
||||
setAnchorEl(null);
|
||||
};
|
||||
|
||||
const exportJson = () => {
|
||||
const jsonString = JSON.stringify(events);
|
||||
const blob = new Blob([jsonString], { type: 'application/json' });
|
||||
const url = URL.createObjectURL(blob);
|
||||
|
||||
const currentDate = new Date().toISOString().split('T')[0];
|
||||
const fileName = `events_${currentDate}.json`;
|
||||
|
||||
const a = document.createElement('a');
|
||||
a.href = url;
|
||||
a.download = fileName;
|
||||
a.click();
|
||||
|
||||
URL.revokeObjectURL(url);
|
||||
setAnchorEl(null);
|
||||
};
|
||||
|
||||
const exportCsv = () => {
|
||||
setAnchorEl(null);
|
||||
};
|
||||
|
||||
return (
|
||||
<StyledActions
|
||||
onClick={(e) => {
|
||||
e.preventDefault();
|
||||
e.stopPropagation();
|
||||
}}
|
||||
>
|
||||
<Tooltip title={'Export'} arrow describeChild>
|
||||
<div>
|
||||
<IconButton
|
||||
aria-label={'Export'}
|
||||
aria-haspopup='true'
|
||||
aria-expanded={open}
|
||||
onClick={handleClick}
|
||||
type='button'
|
||||
>
|
||||
<FileDownload />
|
||||
</IconButton>
|
||||
</div>
|
||||
</Tooltip>
|
||||
<StyledPopover
|
||||
anchorEl={anchorEl}
|
||||
open={open}
|
||||
onClose={handleClose}
|
||||
transformOrigin={{ horizontal: 'right', vertical: 'top' }}
|
||||
anchorOrigin={{ horizontal: 'right', vertical: 'bottom' }}
|
||||
disableScrollLock={true}
|
||||
>
|
||||
<MenuList>
|
||||
<MenuItem onClick={exportCsv}>
|
||||
<ListItemText>
|
||||
<Typography variant='body2'>
|
||||
Export as CSV
|
||||
</Typography>
|
||||
</ListItemText>
|
||||
</MenuItem>
|
||||
<MenuItem onClick={exportJson}>
|
||||
<ListItemText>
|
||||
<Typography variant='body2'>
|
||||
Export as JSON
|
||||
</Typography>
|
||||
</ListItemText>
|
||||
</MenuItem>
|
||||
</MenuList>
|
||||
</StyledPopover>
|
||||
</StyledActions>
|
||||
);
|
||||
};
|
@ -17,6 +17,7 @@ import { EventLogFilters } from './EventLogFilters';
|
||||
import type { EventSchema } from 'openapi';
|
||||
import { useEventLogSearch } from './useEventLogSearch';
|
||||
import { StickyPaginationBar } from 'component/common/Table/StickyPaginationBar/StickyPaginationBar';
|
||||
import { EventActions } from './EventActions';
|
||||
|
||||
interface IEventLogProps {
|
||||
title: string;
|
||||
@ -121,6 +122,7 @@ const NewEventLog = ({ title, project, feature }: IEventLogProps) => {
|
||||
actions={
|
||||
<>
|
||||
{showDataSwitch}
|
||||
<EventActions events={events} />
|
||||
{!isSmallScreen && searchInputField}
|
||||
</>
|
||||
}
|
||||
|
Loading…
Reference in New Issue
Block a user