This PR updates the text used to describe the different fields used in
the new creation dialog.
It also removes a redundant aria attribute (that MUI already handles).
This change prevents long project names from blowing the form out of
proportion.
To do so, it:
1. sets `whitespace: no-wrap` on the button labels. Judging by the other
styles, this was the intention all along, but it didn't really come up
until now.
2. It also sets the label width for projects to 30ch,so that you'll get
to see quite a bit of the project name before it gets cut off.
It would be possible to set a dynamic width for this button based on the
longest project name, but I'm not sure it adds much value, so I'm
leaning towards keeping it simple.
Here's what the dynamic width would look like:
``` tsx
const projectButtonLabelWidth = useMemo(() => {
const longestProjectName = projects.reduce(
(prev: number, type: { name: string }) =>
prev >= type.name.length ? prev : type.name.length,
0,
);
return `${Math.min(longestProjectName, 30)}ch`;
}, [projects]);
```
What it looks like:

This PR makes the config dropdown list generic over its values, so that
you can pass stuff that isn't strings.
It also updates the existing impression data button to use booleans
instead.
This change makes it so that we show the name of the project that is
selected on the selection button instead of the ID. There is a chance
that the name is not unique, but I'm willing to take that risk (plus
it's how we do it today).
I've used a useMemo for this, because we have to scan through a list
to find the right project. Sure, it's always a small list (less than
500 items, I should think), but still nice to avoid doing it every
render. Happy to remove it if you think it obfuscates something.
We *could* also use a `useState` hook and initialize it with the right
value, update when it changes, but I actually think this is a better
option (requires less code and less "remember to update this when that
changes").
This change wraps the project selection option in the
CreateFeatureDialog in a conditional that hides it when Unleash is
OSS.
OSS doesn't have access to the project creation API, so there's no
point in showing this.
This fix validates the project name when you blur the field in the new
project form. The only instances where it'll be wrong is if you have
just whitespace or an empty string, but you'll be notified immediately.
Also removes some unused variables and parameters that I found.
This change makes it so that all form input labels start with a
capital letter, regardless of the data we use to generate them.
Also fixes a leftover toggle -> flag renaming.
This PR extracts the dialog form that we created for the new project
form into a shared component in the `common` folder.
Most of the code has been lifted and shifted, but there's been some
minor adjustments along the way. The main file is
`frontend/src/component/common/DialogFormTemplate/DialogFormTemplate.tsx`.
Everything else is just cleanup.
Add ability to format format event as Markdown in generic webhooks,
similar to Datadog integration.
Closes https://github.com/Unleash/unleash/issues/7646
Co-authored-by: Nuno Góis <github@nunogois.com>
https://linear.app/unleash/issue/2-2469/keep-the-latest-event-for-each-integration-configuration
This makes it so we keep the latest event for each integration
configuration, along with the previous logic of keeping the latest 100
events of the last 2 hours.
This should be a cheap nice-to-have, since now we can always know what
the latest integration event looked like for each integration
configuration. This will tie-in nicely with the next task of making the
latest integration event state visible in the integration card.
Also improved the clarity of the auto-deletion explanation in the modal.
What the title says, adds a subchapter for specs for Unleash, and a
subchapter for specs for the database
---------
Co-authored-by: Nuno Góis <github@nunogois.com>
This PR adds the UI part of feature flag collaborators. Collaborators are hidden on windows smaller than size XL because we're not sure how to deal with them in those cases yet.
https://linear.app/unleash/issue/2-2439/create-new-integration-events-endpointhttps://linear.app/unleash/issue/2-2436/create-new-integration-event-openapi-schemas
This adds a new `/events` endpoint to the Addons API, allowing us to
fetch integration events for a specific integration configuration id.
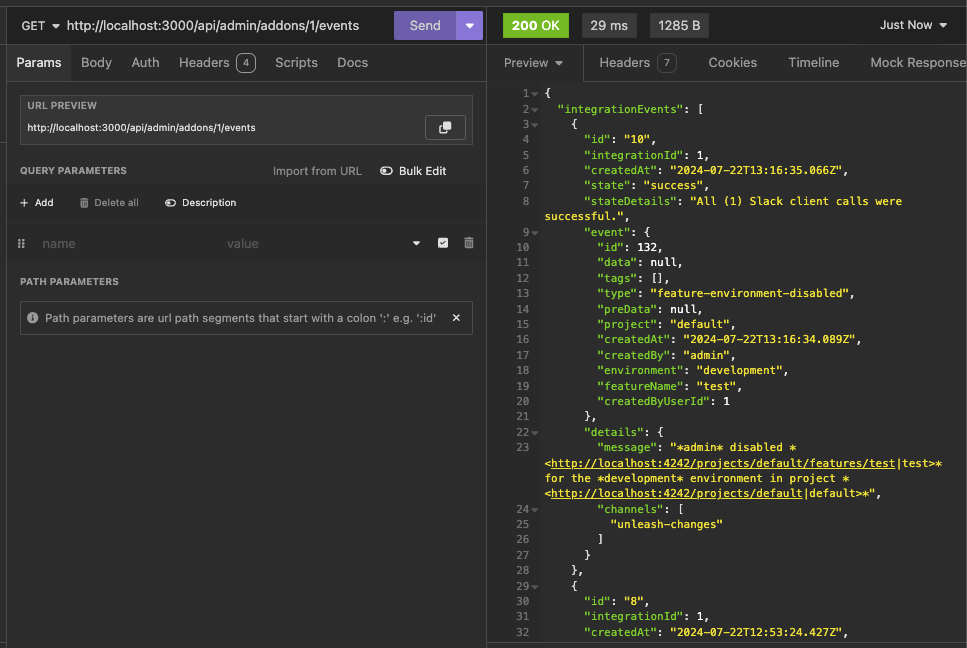
Also includes:
- `IntegrationEventsSchema`: New schema to represent the response object
of the list of integration events;
- `yarn schema:update`: New `package.json` script to update the OpenAPI
spec file;
- `BasePaginationParameters`: This is copied from Enterprise. After
merging this we should be able to refactor Enterprise to use this one
instead of the one it has, so we don't repeat ourselves;
We're also now correctly representing the BIGSERIAL as BigInt (string +
pattern) in our OpenAPI schema. Otherwise our validation would complain,
since we're saying it's a number in the schema but in fact returning a
string.
The limit card says to contact cs@getunleash if you're at the limits,
but we probably don't want to show that to OSS customers (it's not
terrible, just not very helpful), so let's hide it for OSS.
Instead, we'll ask them to try the community slack.
Screenie:

This PR allows you to gradually lower constraint values, even if they're
above the limits.
It does, however, come with a few caveats because of how Unleash deals
with constraints:
Constraints are just json blobs. They have no IDs or other
distinguishing features. Because of this, we can't compare the current
and previous state of a specific constraint.
What we can do instead, is to allow you to lower the amount of
constraint values if and only if the number of constraints hasn't
changed. In this case, we assume that you also haven't reordered the
constraints (not possible from the UI today). That way, we can compare
constraint values between updated and existing constraints based on
their index in the constraint list.
It's not foolproof, but it's a workaround that you can use. There's a
few edge cases that pop up, but that I don't think it's worth trying to
cover:
Case: If you **both** have too many constraints **and** too many
constraint values
Result: You won't be allowed to lower the amount of constraints as long
as the amount of strategy values is still above the limit.
Workaround: First, lower the amount of constraint values until you're
under the limit and then lower constraints. OR, set the constraint you
want to delete to a constraint that is trivially true (e.g. `currentTime
> yesterday` ). That will essentially take that constraint out of the
equation, achieving the same end result.
Case: You re-order constraints and at least one of them has too many
values
Result: You won't be allowed to (except for in the edge case where the
one with too many values doesn't move or switches places with another
one with the exact same amount of values).
Workaround: We don't need one. The order of constraints has no effect on
the evaluation.
https://linear.app/unleash/issue/2-2450/register-integration-events-webhook
Registers integration events in the **Webhook** integration.
Even though this touches a lot of files, most of it is preparation for
the next steps. The only actual implementation of registering
integration events is in the **Webhook** integration. The rest will
follow on separate PRs.
Here's an example of how this looks like in the database table:
```json
{
"id": 7,
"integration_id": 2,
"created_at": "2024-07-18T18:11:11.376348+01:00",
"state": "failed",
"state_details": "Webhook request failed with status code: ECONNREFUSED",
"event": {
"id": 130,
"data": null,
"tags": [],
"type": "feature-environment-enabled",
"preData": null,
"project": "default",
"createdAt": "2024-07-18T17:11:10.821Z",
"createdBy": "admin",
"environment": "development",
"featureName": "test",
"createdByUserId": 1
},
"details": {
"url": "http://localhost:1337",
"body": "{ \"id\": 130, \"type\": \"feature-environment-enabled\", \"createdBy\": \"admin\", \"createdAt\": \"2024-07-18T17: 11: 10.821Z\", \"createdByUserId\": 1, \"data\": null, \"preData\": null, \"tags\": [], \"featureName\": \"test\", \"project\": \"default\", \"environment\": \"development\" }"
}
}
```
This PR updates the limit validation for constraint numbers on a single
strategy. In cases where you're already above the limit, it allows you
to still update the strategy as long as you don't add any **new**
constraints (that is: the number of constraints doesn't increase).
A discussion point: I've only tested this with unit tests of the method
directly. I haven't tested that the right parameters are passed in from
calling functions. The main reason being that that would involve
updating the fake strategy and feature stores to sync their flag lists
(or just checking that the thrown error isn't a limit exceeded error),
because right now the fake strategy store throws an error when it
doesn't find the flag I want to update.