This change fixes a bug where editors were suddenly unable to create
flags. The issue is that the new project creation dialog used a
permission button, but didn't supply the project ID.
This makes the Rust tutorial simpler, by converting to JPEG and Webp,
which means
- Compiles on Ubuntu
- Removes the need for the `rgb` and `ravif` crate
- Makes the `process_images` function a lot simpler
This PR updates the text used to describe the different fields used in
the new creation dialog.
It also removes a redundant aria attribute (that MUI already handles).
This change prevents long project names from blowing the form out of
proportion.
To do so, it:
1. sets `whitespace: no-wrap` on the button labels. Judging by the other
styles, this was the intention all along, but it didn't really come up
until now.
2. It also sets the label width for projects to 30ch,so that you'll get
to see quite a bit of the project name before it gets cut off.
It would be possible to set a dynamic width for this button based on the
longest project name, but I'm not sure it adds much value, so I'm
leaning towards keeping it simple.
Here's what the dynamic width would look like:
``` tsx
const projectButtonLabelWidth = useMemo(() => {
const longestProjectName = projects.reduce(
(prev: number, type: { name: string }) =>
prev >= type.name.length ? prev : type.name.length,
0,
);
return `${Math.min(longestProjectName, 30)}ch`;
}, [projects]);
```
What it looks like:

This PR makes the config dropdown list generic over its values, so that
you can pass stuff that isn't strings.
It also updates the existing impression data button to use booleans
instead.
This change makes it so that we show the name of the project that is
selected on the selection button instead of the ID. There is a chance
that the name is not unique, but I'm willing to take that risk (plus
it's how we do it today).
I've used a useMemo for this, because we have to scan through a list
to find the right project. Sure, it's always a small list (less than
500 items, I should think), but still nice to avoid doing it every
render. Happy to remove it if you think it obfuscates something.
We *could* also use a `useState` hook and initialize it with the right
value, update when it changes, but I actually think this is a better
option (requires less code and less "remember to update this when that
changes").
This change wraps the project selection option in the
CreateFeatureDialog in a conditional that hides it when Unleash is
OSS.
OSS doesn't have access to the project creation API, so there's no
point in showing this.
This fix validates the project name when you blur the field in the new
project form. The only instances where it'll be wrong is if you have
just whitespace or an empty string, but you'll be notified immediately.
Also removes some unused variables and parameters that I found.
This change makes it so that all form input labels start with a
capital letter, regardless of the data we use to generate them.
Also fixes a leftover toggle -> flag renaming.
This PR extracts the dialog form that we created for the new project
form into a shared component in the `common` folder.
Most of the code has been lifted and shifted, but there's been some
minor adjustments along the way. The main file is
`frontend/src/component/common/DialogFormTemplate/DialogFormTemplate.tsx`.
Everything else is just cleanup.
Add ability to format format event as Markdown in generic webhooks,
similar to Datadog integration.
Closes https://github.com/Unleash/unleash/issues/7646
Co-authored-by: Nuno Góis <github@nunogois.com>
https://linear.app/unleash/issue/2-2469/keep-the-latest-event-for-each-integration-configuration
This makes it so we keep the latest event for each integration
configuration, along with the previous logic of keeping the latest 100
events of the last 2 hours.
This should be a cheap nice-to-have, since now we can always know what
the latest integration event looked like for each integration
configuration. This will tie-in nicely with the next task of making the
latest integration event state visible in the integration card.
Also improved the clarity of the auto-deletion explanation in the modal.
What the title says, adds a subchapter for specs for Unleash, and a
subchapter for specs for the database
---------
Co-authored-by: Nuno Góis <github@nunogois.com>
This PR adds the UI part of feature flag collaborators. Collaborators are hidden on windows smaller than size XL because we're not sure how to deal with them in those cases yet.
https://linear.app/unleash/issue/2-2439/create-new-integration-events-endpointhttps://linear.app/unleash/issue/2-2436/create-new-integration-event-openapi-schemas
This adds a new `/events` endpoint to the Addons API, allowing us to
fetch integration events for a specific integration configuration id.
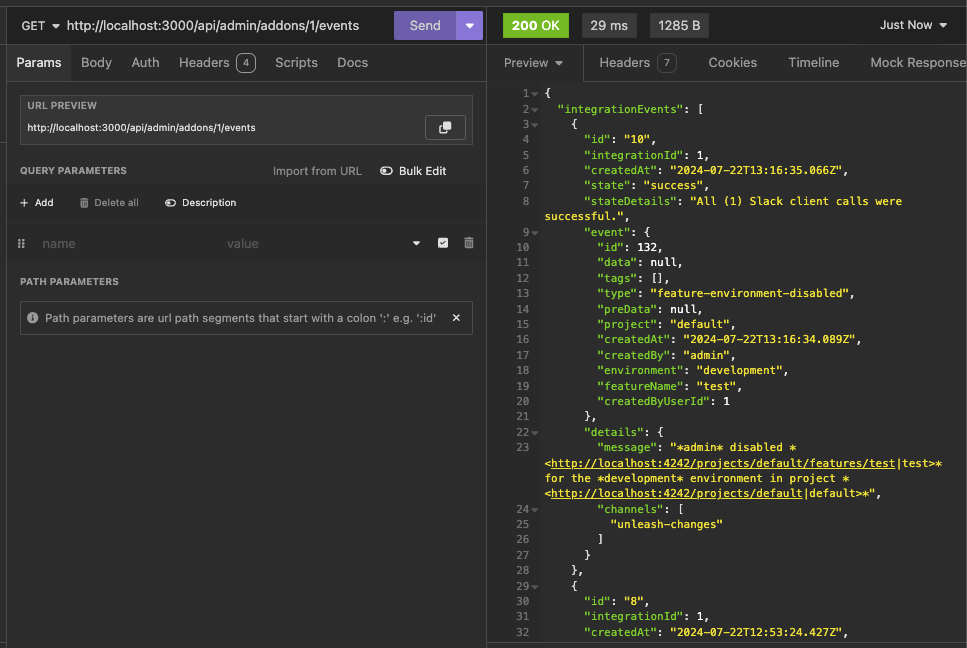
Also includes:
- `IntegrationEventsSchema`: New schema to represent the response object
of the list of integration events;
- `yarn schema:update`: New `package.json` script to update the OpenAPI
spec file;
- `BasePaginationParameters`: This is copied from Enterprise. After
merging this we should be able to refactor Enterprise to use this one
instead of the one it has, so we don't repeat ourselves;
We're also now correctly representing the BIGSERIAL as BigInt (string +
pattern) in our OpenAPI schema. Otherwise our validation would complain,
since we're saying it's a number in the schema but in fact returning a
string.