mirror of
https://github.com/Unleash/unleash.git
synced 2024-10-18 20:09:08 +02:00
https://linear.app/unleash/issue/2-849/dark-mode-ui-fixes 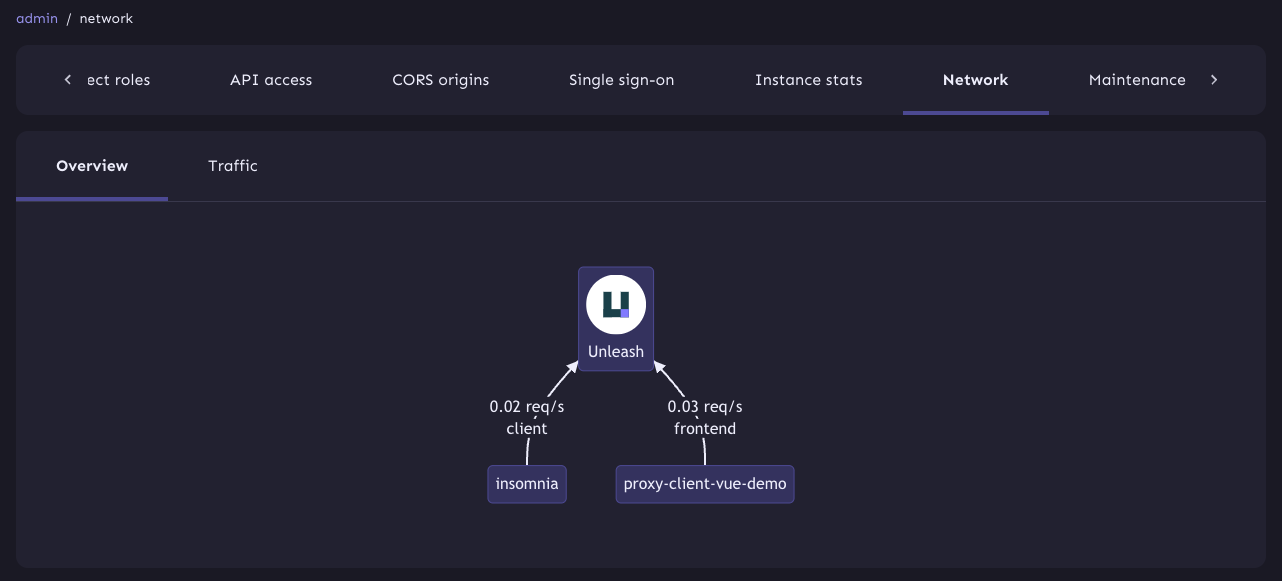 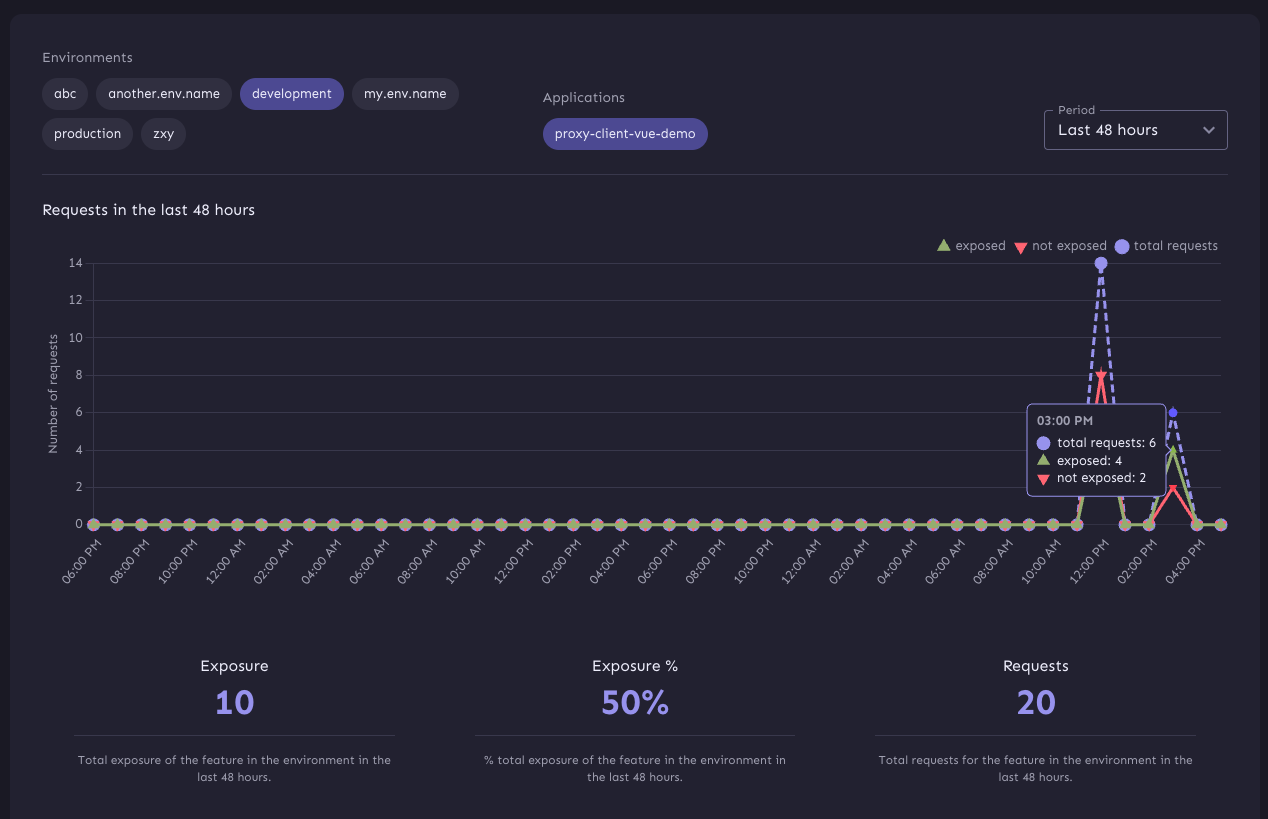 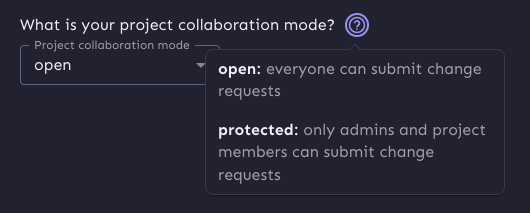 Also includes misc UI fixes and improvements we identified along the way. Co-authored by @NicolaeUnleash --------- Co-authored-by: NicolaeUnleash <103567375+NicolaeUnleash@users.noreply.github.com>
58 lines
1.4 KiB
TypeScript
58 lines
1.4 KiB
TypeScript
import { styled } from '@mui/material';
|
|
import mermaid from 'mermaid';
|
|
import { useRef, useEffect } from 'react';
|
|
|
|
const StyledMermaid = styled('div')(({ theme }) => ({
|
|
display: 'flex',
|
|
justifyContent: 'center',
|
|
'#mermaid': {
|
|
'.edgeLabel': {
|
|
backgroundColor: theme.palette.background.paper,
|
|
color: theme.palette.text.primary,
|
|
},
|
|
'.nodeLabel': {
|
|
color: theme.palette.secondary.dark,
|
|
},
|
|
'.edgePaths > path': {
|
|
stroke: theme.palette.secondary.dark,
|
|
},
|
|
'.arrowMarkerPath': {
|
|
fill: theme.palette.secondary.dark,
|
|
stroke: 'transparent',
|
|
},
|
|
},
|
|
'&&& #mermaid .node rect': {
|
|
stroke: theme.palette.secondary.border,
|
|
fill: theme.palette.secondary.light,
|
|
},
|
|
}));
|
|
|
|
mermaid.initialize({
|
|
startOnLoad: false,
|
|
theme: 'default',
|
|
themeCSS: `
|
|
.clusters #_ rect {
|
|
fill: transparent;
|
|
stroke: transparent;
|
|
}
|
|
`,
|
|
});
|
|
|
|
interface IMermaidProps {
|
|
children: string;
|
|
}
|
|
|
|
export const Mermaid = ({ children, ...props }: IMermaidProps) => {
|
|
const mermaidRef = useRef<HTMLDivElement>(null);
|
|
|
|
useEffect(() => {
|
|
mermaid.render('mermaid', children, svgCode => {
|
|
if (mermaidRef.current) {
|
|
mermaidRef.current.innerHTML = svgCode;
|
|
}
|
|
});
|
|
}, [children]);
|
|
|
|
return <StyledMermaid ref={mermaidRef} {...props} />;
|
|
};
|