mirror of
https://github.com/Unleash/unleash.git
synced 2025-05-08 01:15:49 +02:00
https://linear.app/unleash/issue/2-849/dark-mode-ui-fixes 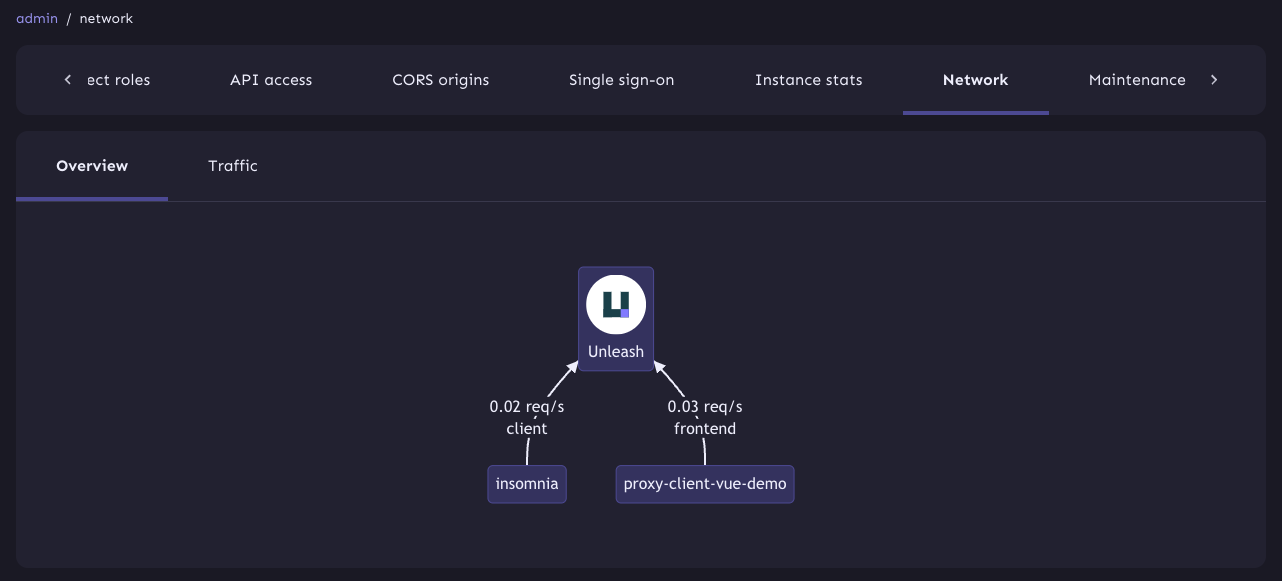 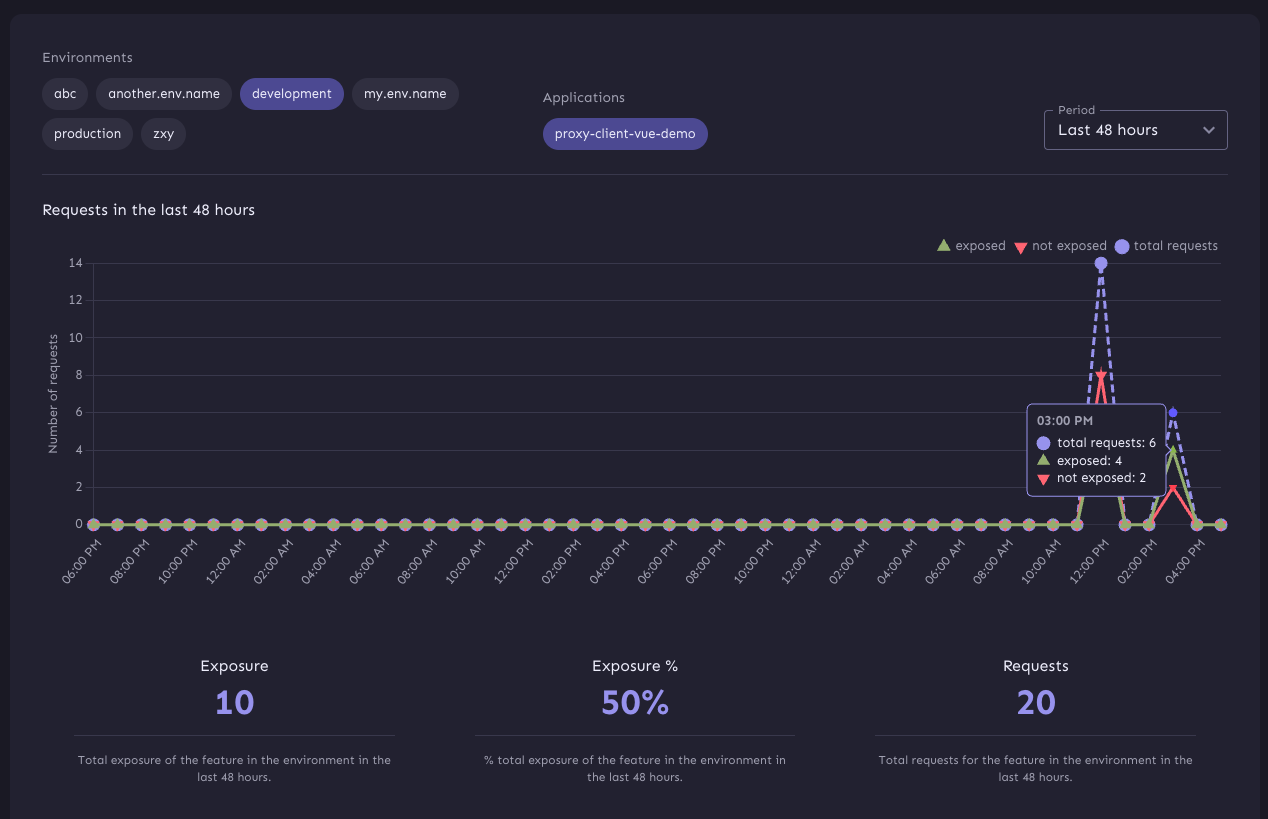 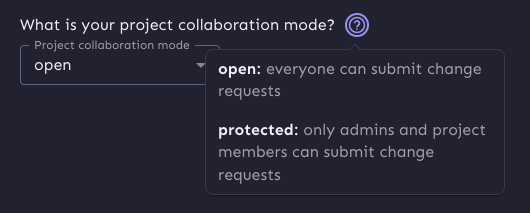 Also includes misc UI fixes and improvements we identified along the way. Co-authored by @NicolaeUnleash --------- Co-authored-by: NicolaeUnleash <103567375+NicolaeUnleash@users.noreply.github.com>
65 lines
2.1 KiB
TypeScript
65 lines
2.1 KiB
TypeScript
import { FeatureMetricsTable } from '../FeatureMetricsTable/FeatureMetricsTable';
|
|
import { IFeatureMetricsRaw } from 'interfaces/featureToggle';
|
|
import { FeatureMetricsStatsRaw } from '../FeatureMetricsStats/FeatureMetricsStatsRaw';
|
|
import { Box, Typography } from '@mui/material';
|
|
import { useId } from 'hooks/useId';
|
|
import React, { Suspense } from 'react';
|
|
|
|
interface IFeatureMetricsContentProps {
|
|
metrics: IFeatureMetricsRaw[];
|
|
hoursBack: number;
|
|
}
|
|
|
|
export const FeatureMetricsContent = ({
|
|
metrics,
|
|
hoursBack,
|
|
}: IFeatureMetricsContentProps) => {
|
|
const statsSectionId = useId();
|
|
const tableSectionId = useId();
|
|
|
|
if (metrics.length === 0) {
|
|
return (
|
|
<Box mt={6}>
|
|
<Typography variant="body1" paragraph>
|
|
We have yet to receive any metrics for this feature toggle
|
|
in the selected time period.
|
|
</Typography>
|
|
<Typography variant="body1" paragraph>
|
|
Please note that, since the SDKs send metrics on an
|
|
interval, it might take some time before metrics appear.
|
|
</Typography>
|
|
</Box>
|
|
);
|
|
}
|
|
|
|
return (
|
|
<Suspense fallback={null}>
|
|
<Box borderTop={1} pt={2} mt={3} borderColor="divider">
|
|
<LazyFeatureMetricsChart
|
|
metrics={metrics}
|
|
hoursBack={hoursBack}
|
|
statsSectionId={statsSectionId}
|
|
/>
|
|
</Box>
|
|
<Box mt={4}>
|
|
<FeatureMetricsStatsRaw
|
|
metrics={metrics}
|
|
hoursBack={hoursBack}
|
|
statsSectionId={statsSectionId}
|
|
tableSectionId={tableSectionId}
|
|
/>
|
|
</Box>
|
|
<Box mt={4}>
|
|
<FeatureMetricsTable
|
|
metrics={metrics}
|
|
tableSectionId={tableSectionId}
|
|
/>
|
|
</Box>
|
|
</Suspense>
|
|
);
|
|
};
|
|
|
|
const LazyFeatureMetricsChart = React.lazy(
|
|
() => import('../FeatureMetricsChart/FeatureMetricsChart')
|
|
);
|