mirror of
https://github.com/Unleash/unleash.git
synced 2025-07-02 01:17:58 +02:00
https://linear.app/unleash/issue/2-811/ability-to-move-project-specific-segments 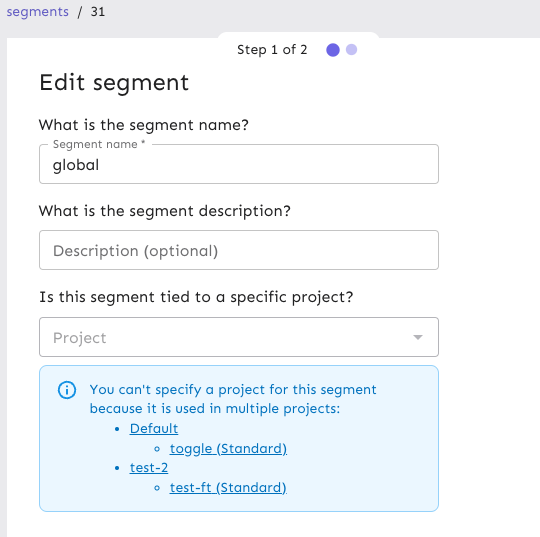 Provides some safeguards to project-specific segments when managing them on a global level (segments page): - You can always promote a segment to global; - You can't specify a project if the segment is currently in use in multiple projects; - You can't specify a different project if the segment is currently already in use in a project;
96 lines
3.2 KiB
TypeScript
96 lines
3.2 KiB
TypeScript
import { Alert, styled } from '@mui/material';
|
|
import { formatEditStrategyPath } from 'component/feature/FeatureStrategy/FeatureStrategyEdit/FeatureStrategyEdit';
|
|
import { IProjectCard } from 'interfaces/project';
|
|
import { IFeatureStrategy } from 'interfaces/strategy';
|
|
import { Link } from 'react-router-dom';
|
|
import { formatStrategyName } from 'utils/strategyNames';
|
|
|
|
const StyledUl = styled('ul')(({ theme }) => ({
|
|
listStyle: 'none',
|
|
paddingLeft: 0,
|
|
}));
|
|
|
|
const StyledAlert = styled(Alert)(({ theme }) => ({
|
|
marginTop: theme.spacing(1),
|
|
}));
|
|
|
|
interface ISegmentProjectAlertProps {
|
|
projects: IProjectCard[];
|
|
strategies: IFeatureStrategy[];
|
|
projectsUsed: string[];
|
|
availableProjects: IProjectCard[];
|
|
}
|
|
|
|
export const SegmentProjectAlert = ({
|
|
projects,
|
|
strategies,
|
|
projectsUsed,
|
|
availableProjects,
|
|
}: ISegmentProjectAlertProps) => {
|
|
const projectList = (
|
|
<StyledUl>
|
|
{Array.from(projectsUsed).map(projectId => (
|
|
<li key={projectId}>
|
|
<Link to={`/projects/${projectId}`} target="_blank">
|
|
{projects.find(({ id }) => id === projectId)?.name ??
|
|
projectId}
|
|
</Link>
|
|
<ul>
|
|
{strategies
|
|
?.filter(
|
|
strategy => strategy.projectId === projectId
|
|
)
|
|
.map(strategy => (
|
|
<li key={strategy.id}>
|
|
<Link
|
|
to={formatEditStrategyPath(
|
|
strategy.projectId!,
|
|
strategy.featureName!,
|
|
strategy.environment!,
|
|
strategy.id
|
|
)}
|
|
target="_blank"
|
|
>
|
|
{strategy.featureName!}{' '}
|
|
{formatStrategyNameParens(strategy)}
|
|
</Link>
|
|
</li>
|
|
))}
|
|
</ul>
|
|
</li>
|
|
))}
|
|
</StyledUl>
|
|
);
|
|
|
|
if (projectsUsed.length > 1) {
|
|
return (
|
|
<StyledAlert severity="info">
|
|
You can't specify a project for this segment because it is used
|
|
in multiple projects:
|
|
{projectList}
|
|
</StyledAlert>
|
|
);
|
|
}
|
|
|
|
if (availableProjects.length === 1) {
|
|
return (
|
|
<StyledAlert severity="info">
|
|
You can't specify a project other than{' '}
|
|
<strong>{availableProjects[0].name}</strong> for this segment
|
|
because it is used here:
|
|
{projectList}
|
|
</StyledAlert>
|
|
);
|
|
}
|
|
|
|
return null;
|
|
};
|
|
|
|
const formatStrategyNameParens = (strategy: IFeatureStrategy): string => {
|
|
if (!strategy.strategyName) {
|
|
return '';
|
|
}
|
|
|
|
return `(${formatStrategyName(strategy.strategyName)})`;
|
|
};
|