mirror of
https://github.com/Unleash/unleash.git
synced 2024-11-01 19:07:38 +01:00
https://linear.app/unleash/issue/2-849/dark-mode-ui-fixes 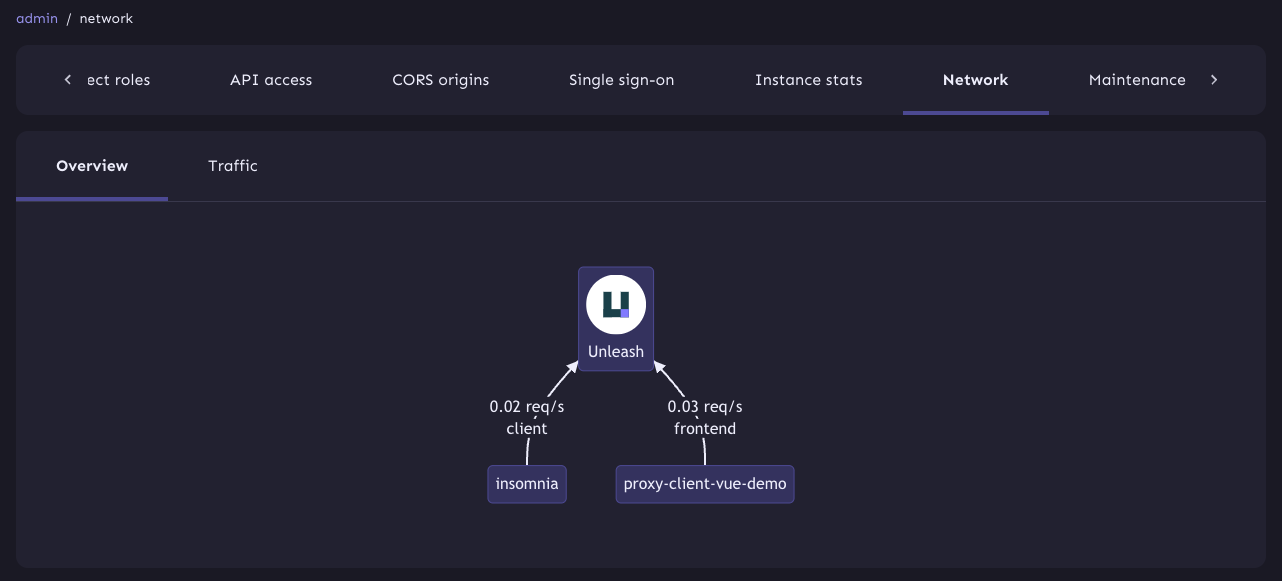 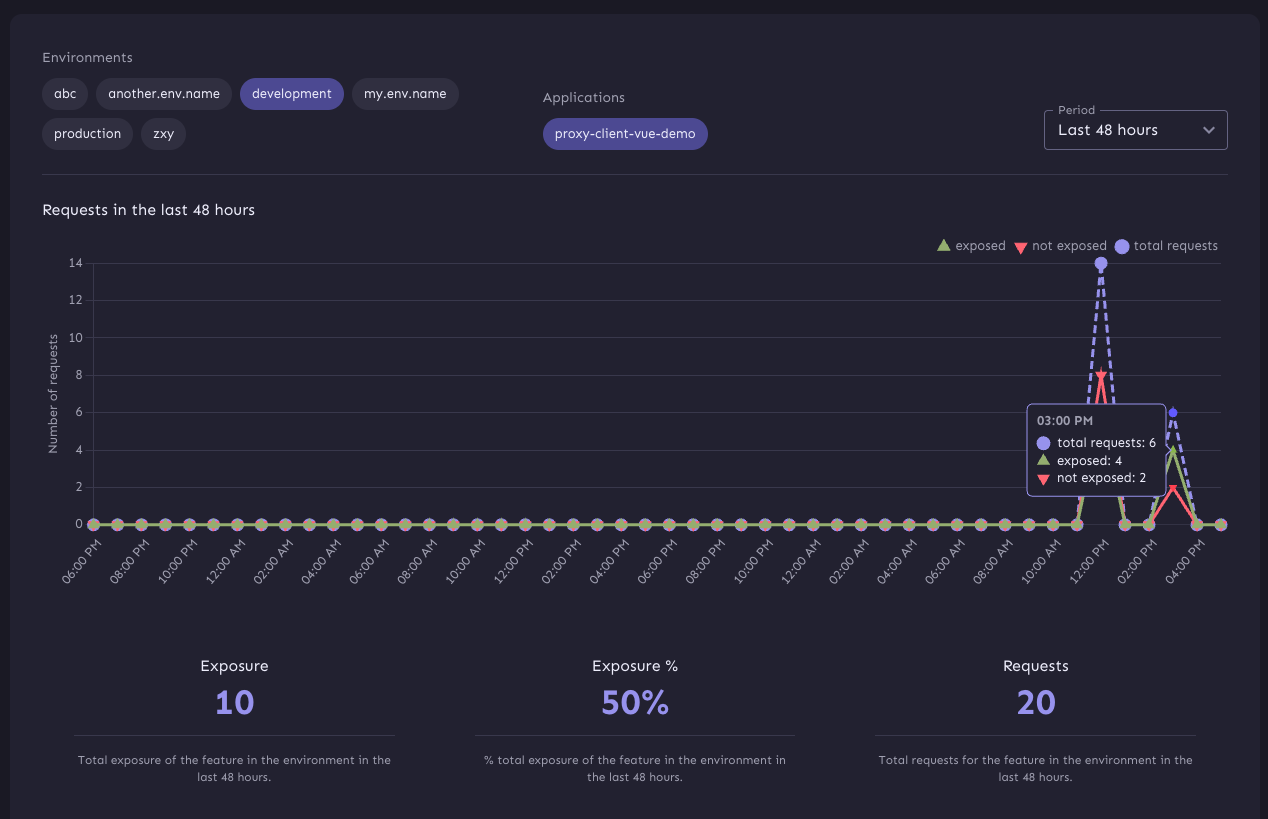 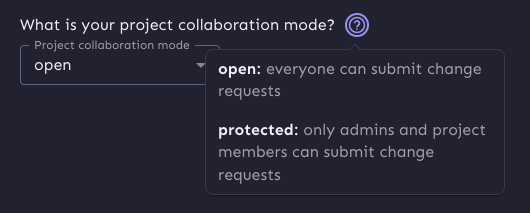 Also includes misc UI fixes and improvements we identified along the way. Co-authored by @NicolaeUnleash --------- Co-authored-by: NicolaeUnleash <103567375+NicolaeUnleash@users.noreply.github.com>
39 lines
1.0 KiB
TypeScript
39 lines
1.0 KiB
TypeScript
import UIContext, { themeMode } from 'contexts/UIContext';
|
|
import { useContext } from 'react';
|
|
import { setLocalStorageItem } from 'utils/storage';
|
|
import mainTheme from 'themes/theme';
|
|
import darkTheme from 'themes/dark-theme';
|
|
import { Theme } from '@mui/material/styles/createTheme';
|
|
|
|
interface IUseThemeModeOutput {
|
|
resolveTheme: () => Theme;
|
|
onSetThemeMode: () => void;
|
|
themeMode: themeMode;
|
|
}
|
|
|
|
export const useThemeMode = (): IUseThemeModeOutput => {
|
|
const { themeMode, setThemeMode } = useContext(UIContext);
|
|
const key = 'unleash-theme';
|
|
|
|
const resolveTheme = () => {
|
|
if (themeMode === 'light') {
|
|
return mainTheme;
|
|
}
|
|
|
|
return darkTheme;
|
|
};
|
|
|
|
const onSetThemeMode = () => {
|
|
setThemeMode((prev: themeMode) => {
|
|
if (prev === 'light') {
|
|
setLocalStorageItem(key, 'dark');
|
|
return 'dark';
|
|
}
|
|
setLocalStorageItem(key, 'light');
|
|
return 'light';
|
|
});
|
|
};
|
|
|
|
return { resolveTheme, onSetThemeMode, themeMode };
|
|
};
|