This change primarily adds all flags to the flag filter and restructures
the filters component. Instead of splitting into three smaller
components, we now handle more data in the main component.
We might wanna turn them back to smaller components later, but I think
this'll be easier to work with.
Fixes an issue where the collaborator component would be smooshed
together when you have too many collaborators and too many flag tab
items.
The primary things I have done are:
1. Limit the amount of collaborators we show to 6 instead of 8. I
believe the number 8 was arbitrary, so let's go with 6 for now.
2. Instead of using a fixed gap, use a separator element that grows up
to a certain limit. I've added a `Separator` component, which is an
empty div with flex-grow. It feels like you should be able to do that
with gap too, but I can't think of how right now.
3. Don't allow collaborator component text (or avatars) to wrap. We
don't have a lot of space in this header, so let's keep it tight.
Additionally, I've added the `className` prop to the AvatarGroup
component so that it can be styled externally. I also cleaned up some
naming that was left in while I was at it.
Before:

After:

Fixes a bug introduced with the new tooltips where the system user was
shown as "User ID n" instead of "System". The "n" in this case is
actually the user's index number in the list of project owners
(including duplicates).
There's a few things happening:
1. Change the object for system owners: use `name` instead of
`description`. At the same time, remove the `description` property
completely because it's not used at the moment.
2. Remove the assignnment of `id: objectId(user)` to the user sent to
the User Avatar component. This was a leftover from when we split out
the AvatarGroup component, and is not something we use anymore.
Before:

After:

This PR makes some small changes to how we handle strategy deletion in
the demo environment, which has become extra important with the recent
soft limits.
The changes are:
- lower the strategy limit from 30 to 25. The standard limit is 30, so
we want to make sure we're below that.
- when checking whether we should delete a strategy, check whether we're
**at or above** the limit. It used to only check if we were above, but
if soft limits would prevent you from adding more, then you'd never be
able to go above the limit.
- Also delete strategies for step3.
Old versions of Unleash allow for creating "Gradual Rollout" strategies
without `groupId` or `stickiness`. UI will now populate those fields,
not getting stuck when editing strategies without said fields.
Fixes an issue where the filter buttons were both too far down and too
far to the right.
The issue was that the wrapper body imposed a pretty substantial bit
of padding. However, the filter buttons already came with their own
bit of padding. The result of this was alignment issues.
To fix it I have:
- opened the `Filters` component up to be styled with styled components
And conditionally (when isEnterprise and the flag is on):
- set the page body to have no padding.
- added a wrapper with padding around the event search results for
This feels a little messy to me, but I also think that because it's
still in heavy development, it might change later. I'd be happy to have
suggestions forbetter implementations.
What makes this extra tricky is that the top padding differs depending
on whether you have the filters there or not, so I couldn't find a way
to just remove that component and be done with it. I may very well have
missed somehing, though.
Before:

After:

Changes the type used by the useEventSearch hook to be `EventSchema`
from OpenAPI instead. This is more accurate with what we're actually
getting. And crucially for the event log search, it contains the
`createdByUserId` property that we need to filter out events.
It's mostly a straightforward find and replace except for one instance
where we need to do some extra fiddling. There's an inline comment
explaining that.
Extracts the Avatar Group component into a `common` component and adds a
standard tooltip to all avatars.
Relates to linear issue 1-2606
This is a suggestion / proof of concept for how we can solve it. While I
think we can merge this as is, I'd also be happy to take any discussions
on other ways to approach it etc.
## Why are these changes made together?
Because extracting the avatar group without adding the new tooltip data
made the existing tooltip misbehave (it'd show up in the top left of the
screen, not synced to the avatar in any way).
I probably could have (and still can if you think it's prudent) split it
out such that the avatar gets a standardized tooltip first (and disable
it for the group card avatars), and split out the avatars in a
follow-up. Happy to do that if you think it's better.
## What does this mean?
It used to be that we had no consistent way of dealing with avatars and
tooltips. Some places had them, some places didn't. This change makes it
so that all avatars that we can show tooltips for will get the same
tooltip.
Previously, we had at least 4 different ways of dealing with tooltips:
- The HTML tooltip (that would be standardized with this PR) in the
project flags table

- The "title" that you'd get on your user avatar
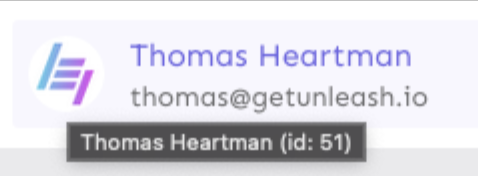
- The group card list tooltip

- And sometimes you'd get nothing at all

with this change, we'll always show the same kind of tooltip if we can:

## What goes in the tooltip?
We use the `UserAvatar` component for a fair few different things and I
didn't want to extract separate components for all the different use
cases. Instead, I wanted to get an overview over what we use it for and
what is relevant info to show.
I found all the places we used it and tried to form an opinion.
This tooltip will work with a user's email, name, username, and id. If
there is no user (such as for empty avatars and avatars displaying only
"+n" for remaining members), we show no tooltip.
Following the example set by the group card avatars, we'll try to use
email or username (in that order) as the main bit of text. If the user
has an email or a username and also a name, the name will be used as
secondary text.
If the user does not have an email or username, but has a name, we'll
use the name as the main text.
If the user does not have an email, a username, or a name, we'll try to
show "User ID: N" if they have an id.
If they do not have a username, a name, an email, or an ID, we bail out
and show nothing.
## Why can you disable the tooltip?
In some cases, you might want to disable the tooltip because you have
more information to feed into it. An example of that is in the project
flags table, where we want to show more information in cases where the
user is 'unknown':

## Additional fixes
This PR also adds a few lines of CSS to fix a minor avatar layout bug.
Before:
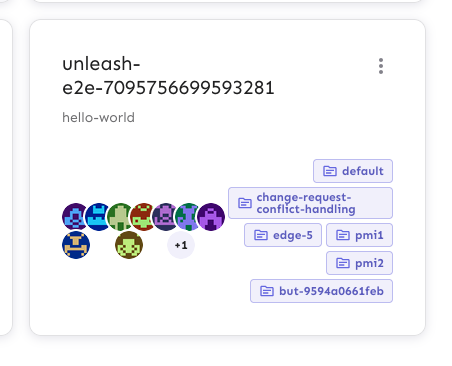
After:

Adds placeholder filter buttons (that don't work at all) yet to the
three event logs.
Flag logs get to choose to and from dates, created by, and event type.
Project logs get all that flag logs get + a filter for flag.
The global log gets all project log filters + a project filter.
There's still work to be done to add data to createdBy, eventType, to
hook it up to the API, and to finalize the layout, but I wanted to get a
rough outline in to iterate on later. The eventType icon will also need
to be decided on.

This PR fixes a minor visual issue where the "favorite project" button's
hover outline would get cut off due to its container having `overflow:
hidden`.
The overflow value was introduced in
[#7575](https://github.com/Unleash/unleash/pull/7575) as way to handle
long project names. We didn't discover the hover issue back then because
it's not apparent unless you hover the fav star.
I found the solution in the CSS-tricks post [Preventing a Grid
Blowout](https://css-tricks.com/preventing-a-grid-blowout/). To quote
the article, the reason this works is that:
> the minimum width of a grid column is auto. (The same is true for flex
items, by the way.)
>
> And since auto is entirely based on content, we can say it is
“indefinitely” sized, its dimensions flex. If we were to put an explicit
width on the column, like 50% or 400px, then we would say it is
“definitely” sized.
>
> To apply our fix, we need to make sure that there is the column has a
definite minimum width instead of auto.
Before:

After:

Additionally, I've removed a duplicate declaration of font size,
removing the deprecated version.
This change fixes a bug where editors were suddenly unable to create
flags. The issue is that the new project creation dialog used a
permission button, but didn't supply the project ID.
This PR updates the text used to describe the different fields used in
the new creation dialog.
It also removes a redundant aria attribute (that MUI already handles).