Extracts each panel into its own component for the personal dashboard.
This lets us use separate states for each panel, which in turn lets each
panel change its open / close state without causing the other panels to
re-render.
When you have a lot of flags and/or projects, the list to render becomes
very long, which causes performance problems, especially when you need
to rerender both flags and projects and the timeline whenever one of
them changes.
The problems were especially noticeable in Firefox for me. Even with
this, the event timeline is a little choppy. I suspect that's because of
it might take a long time to paint? But we can look into that later.
Also updates the dashboard state hook to let you only pass in the
flags/projects you want. We could extract this into three different
hooks that all use the same localhost key, but I'm not sure whether
that's better or worse 🤷🏼
Prevents tab from navigating you through the list of results. Instead
makes it so that the tab key always takes you to the next item in the
same hierarchy.
As a bonus: also automatically closes the menu when you navigate
away (the previous implementation has a bug where it wouldn't if you
shift-tab).
The behavior of not letting you navigate the list with tab is
consistent with native HTML select elements as well as MUI select
elements. You typically navigate them with the arrow keys.
https://linear.app/unleash/issue/2-2834/plausible
Adds the following Plausible events to the Release management feature:
- Add plan
- Start milestone
- Remove plan
- Create template
- Edit template
- Delete template
Moves the event timeline to the personal dashboard from the header when
the `frontendHeaderRedesign` flag is active.
When the flag is active, it also:
- hides the event timeline and corresponding button in the header
- renders the environment selector next to the time selector instead of
at the other end of the header
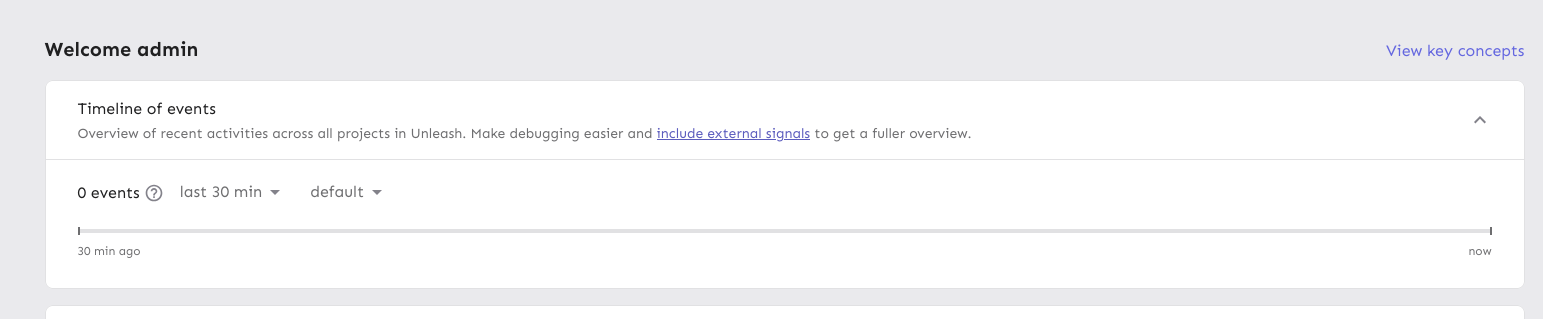
---------
Co-authored-by: Nuno Góis <github@nunogois.com>
Fixes the issue where the skip link wouldn't take you to the main
content of the page anymore.
Also includes a few related minor semantic and a11y improvements:
1. The `main` element now only surrounds the actual main content of the
page. The sidebar is nav content and shouldn't be within it. The easiest
way to do this was to change the element that was previously a `main` to
a `div` and make the main content wrapper a `main` instead.
2. Makes the skip link target visible when focused. But invisible
otherwise. This has two benefits:
1. It's immediately obvious that using the skip link has worked. It
tells you that it's at the start of the main content.
2. Because the link now has text, it can be targeted by link search
(e.g. in Firefox, press `'` to search for links (I use this **a lot**)),
making it super easy to move your focus to the main content directly.
(Yes, landmark navigation should also work here, though, especially with
the `main` change).
The implementation of UI considerations of the skip link are based on
the CSS-tricks article [a deep dive on skipping to
content](https://css-tricks.com/a-deep-dive-on-skipping-to-content/)
from 2021.
Here's what it looks like when you skip to content:

When it doesn't have focus, it's invisible.
Makes the data returned from the traffic search a union type to avoid
nasty object-is-undefined errors at runtime.
It requires more explicit handling, sure, but it means we don't need
to accept undefined.
Adds new monthly estimation functions that operate on raw usage data
instead of chart data. This brings those methods in line with the rest
of the traffic calculation functions that we have in that file and means
we can remove other external dependencies.
This is somewhat inspired by #9218, but not directly linked.
Updates the existing BillingDetails pages (pro and payg) to use the new
traffic search endpoint (and calculations) if the flag to do so is on.
Otherwise, it falls back to using the existing method.
I've extracted the overage calculation into a separate shared hook.
Implements a function that cleans and filters incoming data from the
new traffic API.
Specifically, it:
- Removes `/edge` data points
- Removes any data from before may 2024, which is the first full month
we have on record
Because all uses of the existing hook do this filtering themselves, I
have added the filtering at the hook level. This is to avoid
forgetting this filtering later. If we find out we need this data, we
can move the filtering.
Refactors the period selector component now that the design / system is
pretty much finished.
Main points are: change from using CSS selectors to using styled
components; use props instead of classes. This is in keeping with the
general Unleash approach.
There's two very slight visual changes here:
1. There is 4px of added space below the "range" "header" text.
2. The months in the grid are a little closer together and not as wide.
This is because we remove the explicit column gap due to the grid having
a set width. Previously the width was automatic, but because we want
this to line up with the button, we need to set the width explicitly on
both items. As such, with the padding, the grid was a little too wide,
so there was too little padding on the right. This rectifies that.
This PR refactors the `NetworkTrafficUsage.tsx` and `useTrafficData`
files a bit.
The primary objective was to make the network traffic usage component
easier to work with, so I suggest to the reviewer that they start there.
Part of that refactoring, was taking things out of the useTraffic hook
that didn't need to be there. In the end, I'd removed so much that I
didn't even need the hook itself in the new component, so I switched
that to a regular useState.
It made more sense to me to put some of the functions inside the hook
into a separate file and import them directly (because they don't rely
on any hook state), so I have done that and removed those functions from
the trafficData hook. In this case, I also moved the tests.
I have not added any new tests in this PR, but will do so in a
follow-up. The functions I intend to test have been marked as such.
## About the changes
This gives us the ability to communicate other license messages which
are not errors. By default they'll be warning but I'm opening the
possibility of using a backend-provided value to make them informative
instead of warning.
The intention is to communicate things like:
- Your license is about to expire in x days.
- You are getting close to the maximum number of seats in your license
- etc
The test was breaking because it assumed a month would have at least
30 days.
Because the test relies on the current month, this isn't necessarily
true.
Further, there's parts of the code that relies on "impure" state via
the "current date" (which will change based on when you run it), so
setting a specific month in the test won't work.
As such, this test makes the calculation explicit and uses the number
of days in the current month.
Wraps the datepicker in a popover, making it function largely the same
as a dropdown list.
The dropdown displays one of:
- "current month" if you've selected the current month
- "<month> <year>" (e.g. "December 2024") if you've selected a month
that isn't the current month
- "Last n months" (e.g. "Last 3 months") if you have selected a range
Additionally, the range selections have been updated to span the whole
row, aligning with the look of generic dropdown lists.
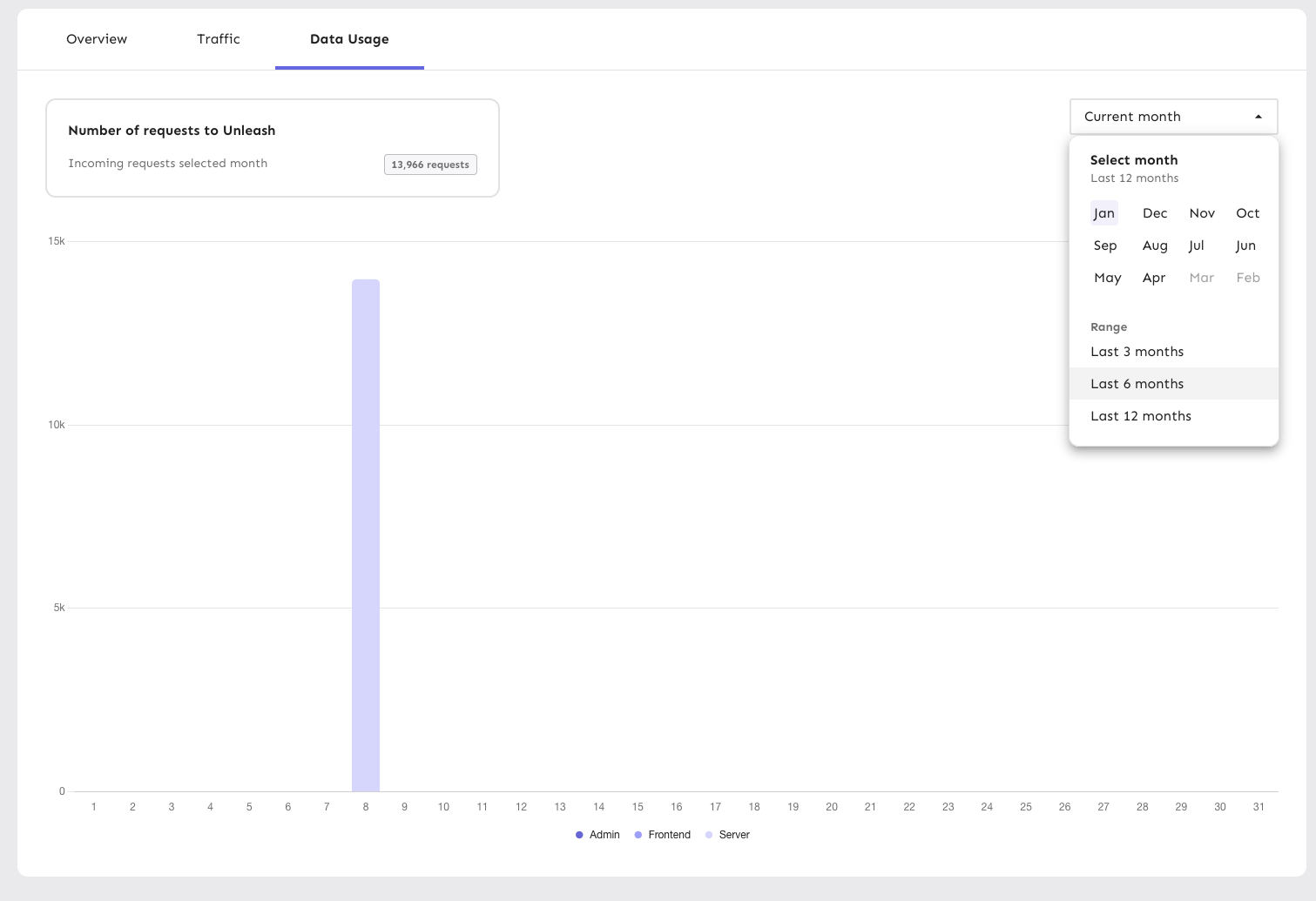
Like with the rest of this file (`PeriodSelector`), the code is rough
and not according to Unleash standards. However, I'm prioritizing fast
changes so UX can have a look before I clean up the code to switch to
using styled components etc later. It's still behind a flag, so I'm not
very worried about it.
This makes the width of the highlight bars in the network dynamic and
based on the number of labels included in the chart.
Since the number of labels should always correspond to the number of
data points, this seems like a sensible approach.
With this, the label width will now be calculated on the fly, so even if
you resize the window or change the number of labels, the highlighting
will still work as expected.
Daily view:
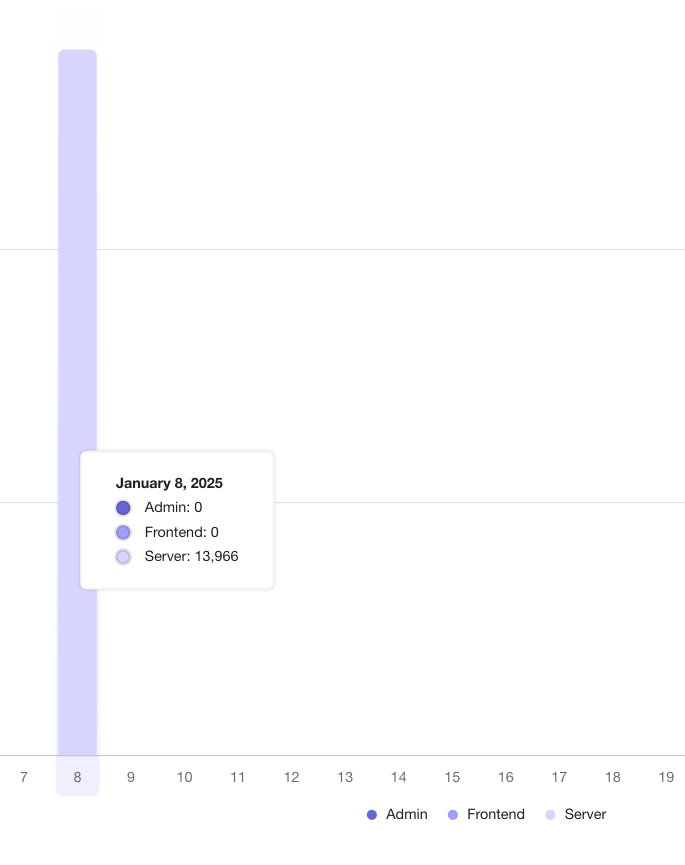
Monthly aggregate:
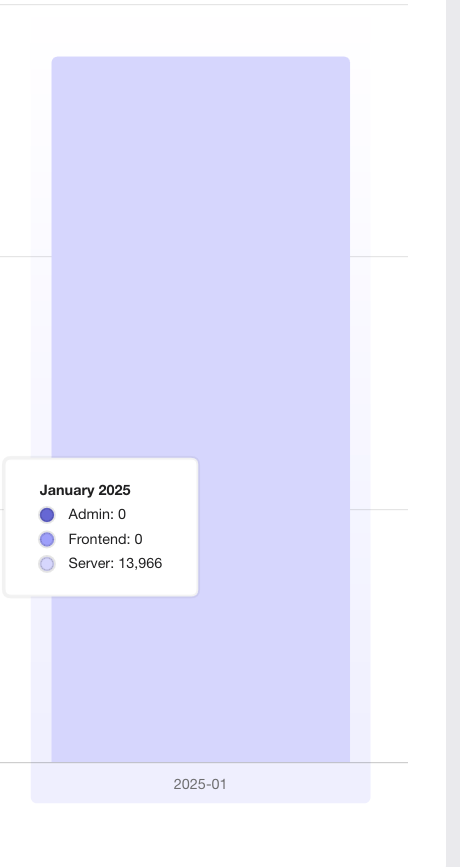
The labels are now a little narrower on the daily graphs, but it avoids
them being super wide on the monthly graphs
Adds support for the new /traffic-search API behind a flag. When active, you'll be able to select month ranges as well as specific single months.
Largely copies the existing network traffic component, and adds some minor tweaks to make it work with the new data.
This is quite rough, but it gives us a base to build on for later. There's still things that we need to solve for in following PRs.
This PR sets up the application to accept a value from a variant we
control to set the font size of the application on a global level. If it
fails, the value falls back to the previously set CSS value.
This PR implements a first version of the new month/range picker for the
data usage graphs. It's minimally hooked up to the existing
functionality to not take anything away.
This primary purpose of this PR is to get the design and interaction out
on sandbox so that UX can have a look and we can make adjustments.
As such, there are a few things in the code that we'll want to clean up
before removing the flag later:
- for faster iteration, I've used a lot of CSS nesting and element
selectors. this isn't usually how we do it here, so we'll probably want
to extract into styled components later
- there is a temporary override of the value in the period selector so
that you can select ranges. It won't affect the chart state, but it
affects the selector state. Again, this lets you see how it acts and
works.
- I've added a `NewHeader` component because the existing setup smushed
the selector (it's a MUI grid setup, which isn't very flexible). I don't
know what we want to do with this in the end, but the existing chart
*does* have some problems when you resize your window, at least
(although this is likely due to the chart, and can be solved in the same
way that we did for the personal dashboards).
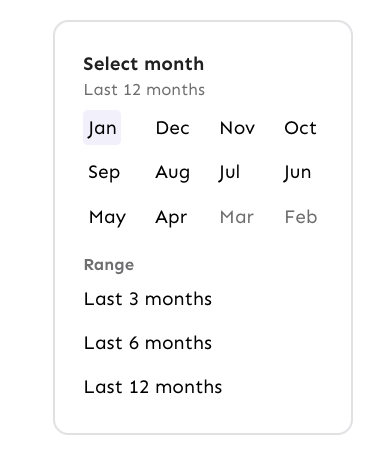