mirror of
https://github.com/Unleash/unleash.git
synced 2025-01-06 00:07:44 +01:00
This change fixes an issue with the loader where it would explode its parent component to 100vh even when that was not called for. To do so, I've added the a new `type` prop to the component, to distinguish between `fullscreen` and `inline` usage. The `fullscreen` type sets the height to 100vh, while the `inline` type sets it to 100%. Now, this doesn't directly make the loader fullscreen (it just makes sure it's at least as tall as the screen), so maybe the prop name is misleading. I'd be happy to change it (or to even extract this into two separate components) if that's preferable. Other potential prop names could be `height`, which is very direct, or `usage`, which I think better describes what we do. Like with `type`, I'd like to communicate the intended behavior more that the actual implementation, so I'm leaning towards either `type` or `usage`. ## Screenies I've gone through all the usages of the loader, and checked how each one works. Here they are: ### Loader in environment variants I wasn't able to trigger this manually, but it's apparently there Old (ignore the banner placement; that's firefox's screenshot tool acting up)  New: 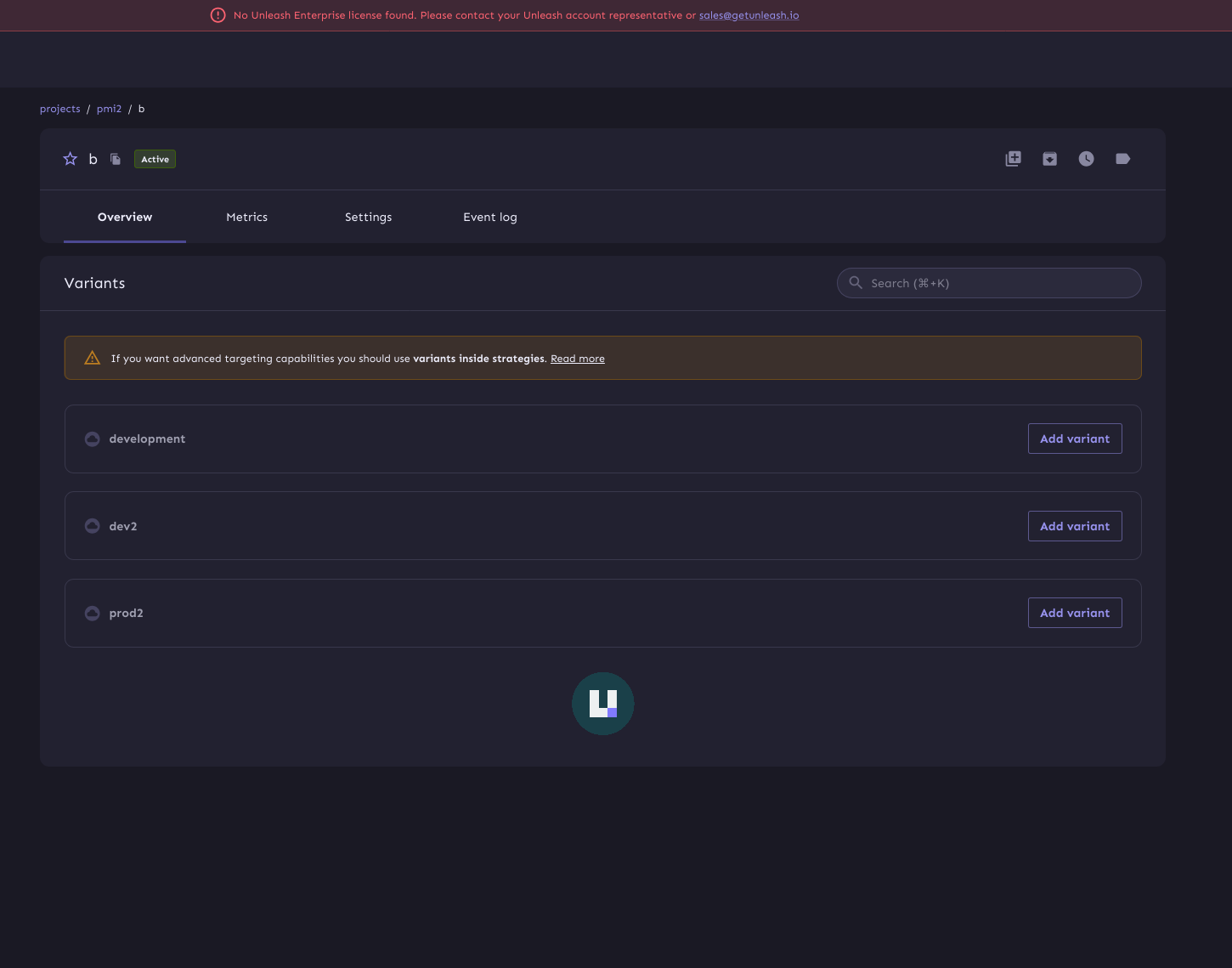 ### Project setting forms Old: 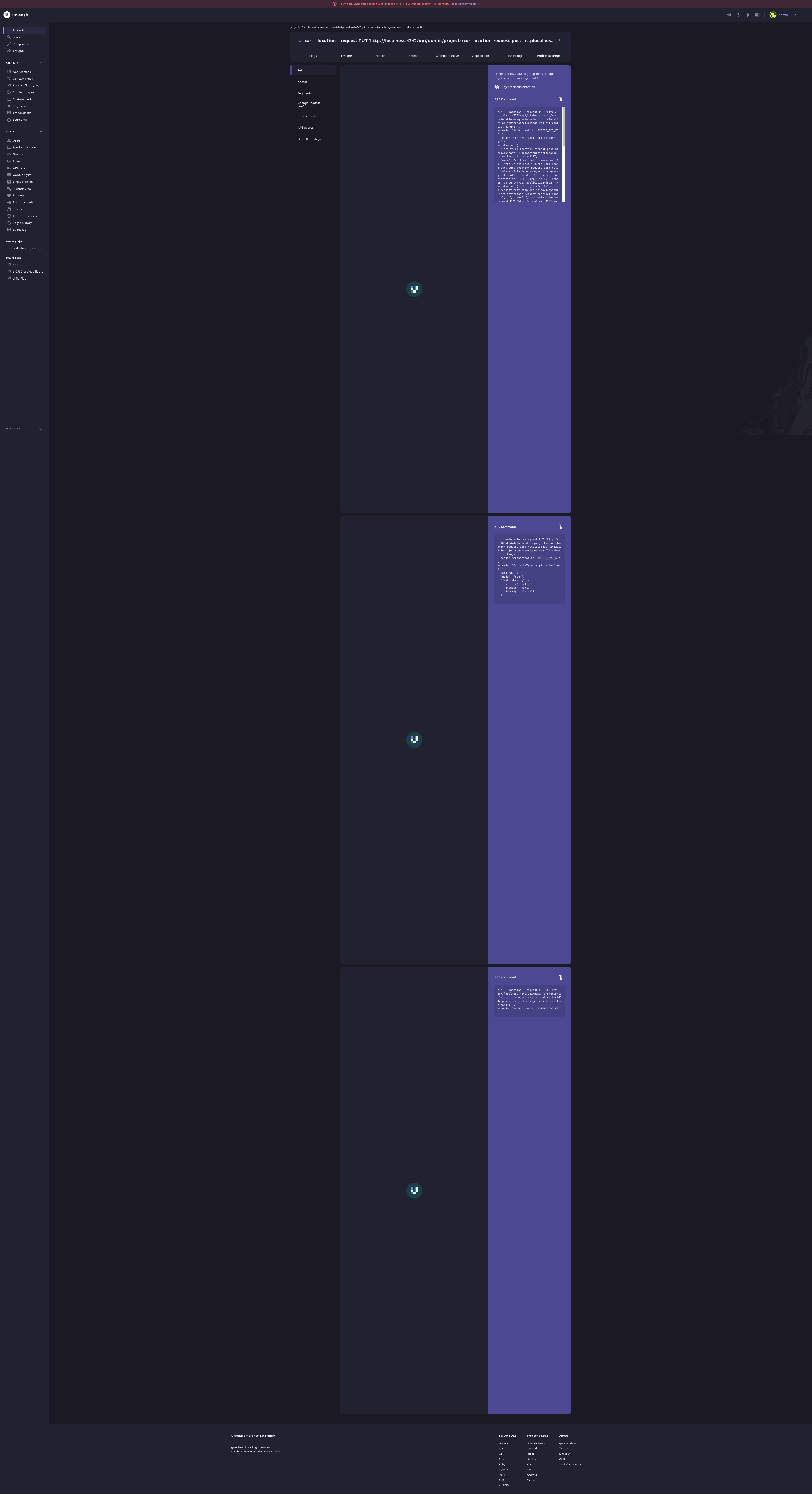 New: 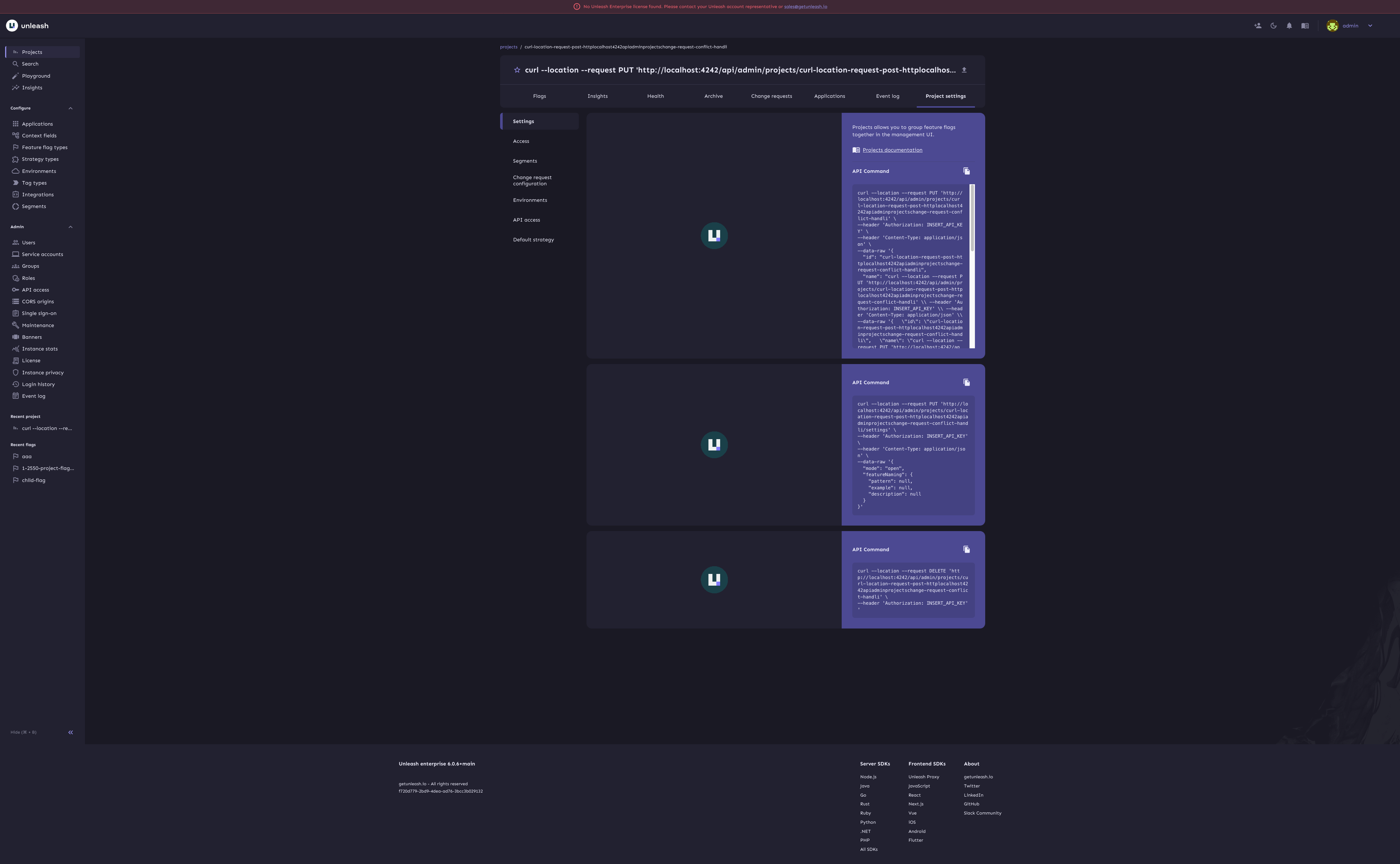 ### Rollout strategy Old: 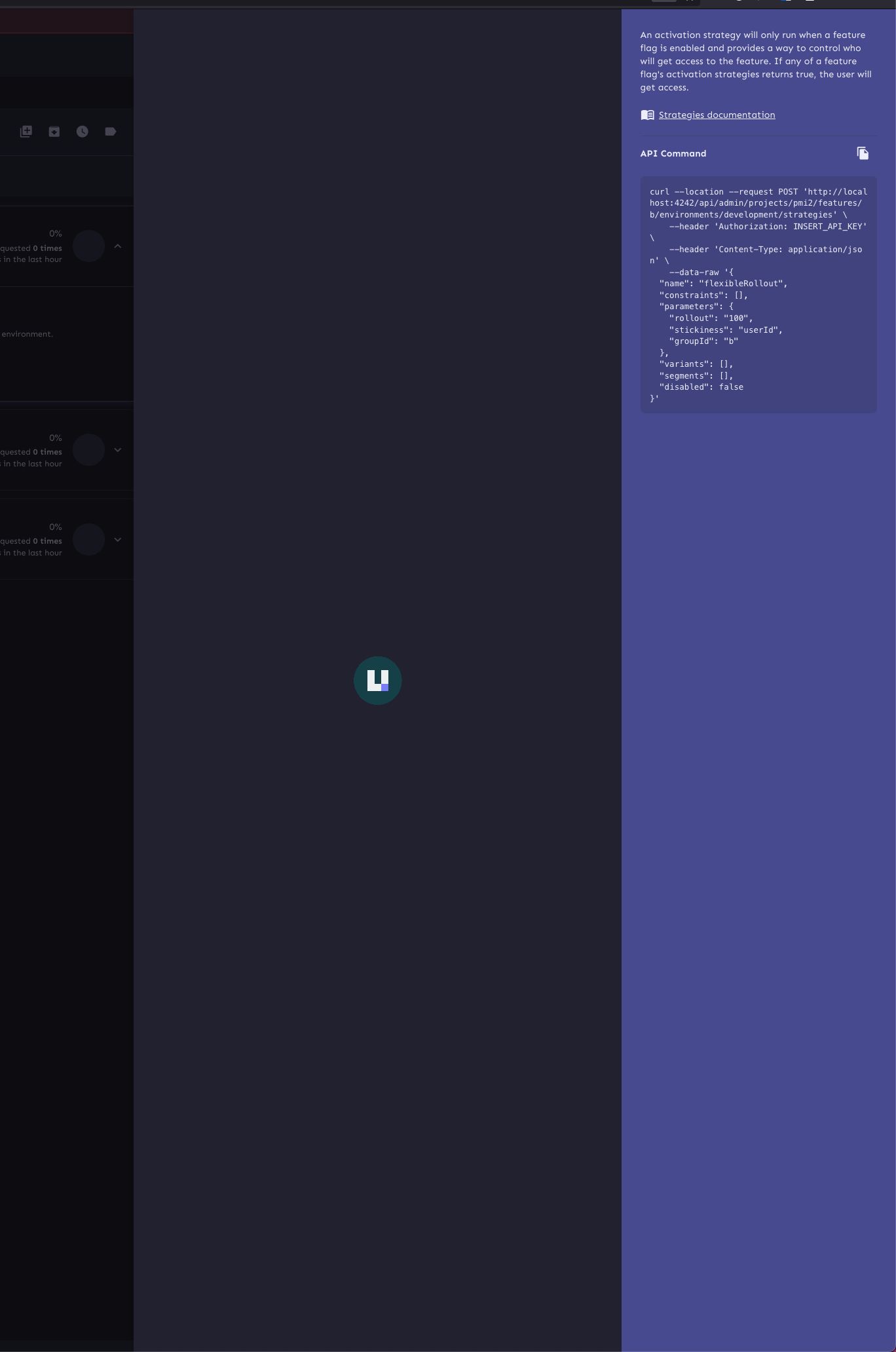 New (no discernible change): 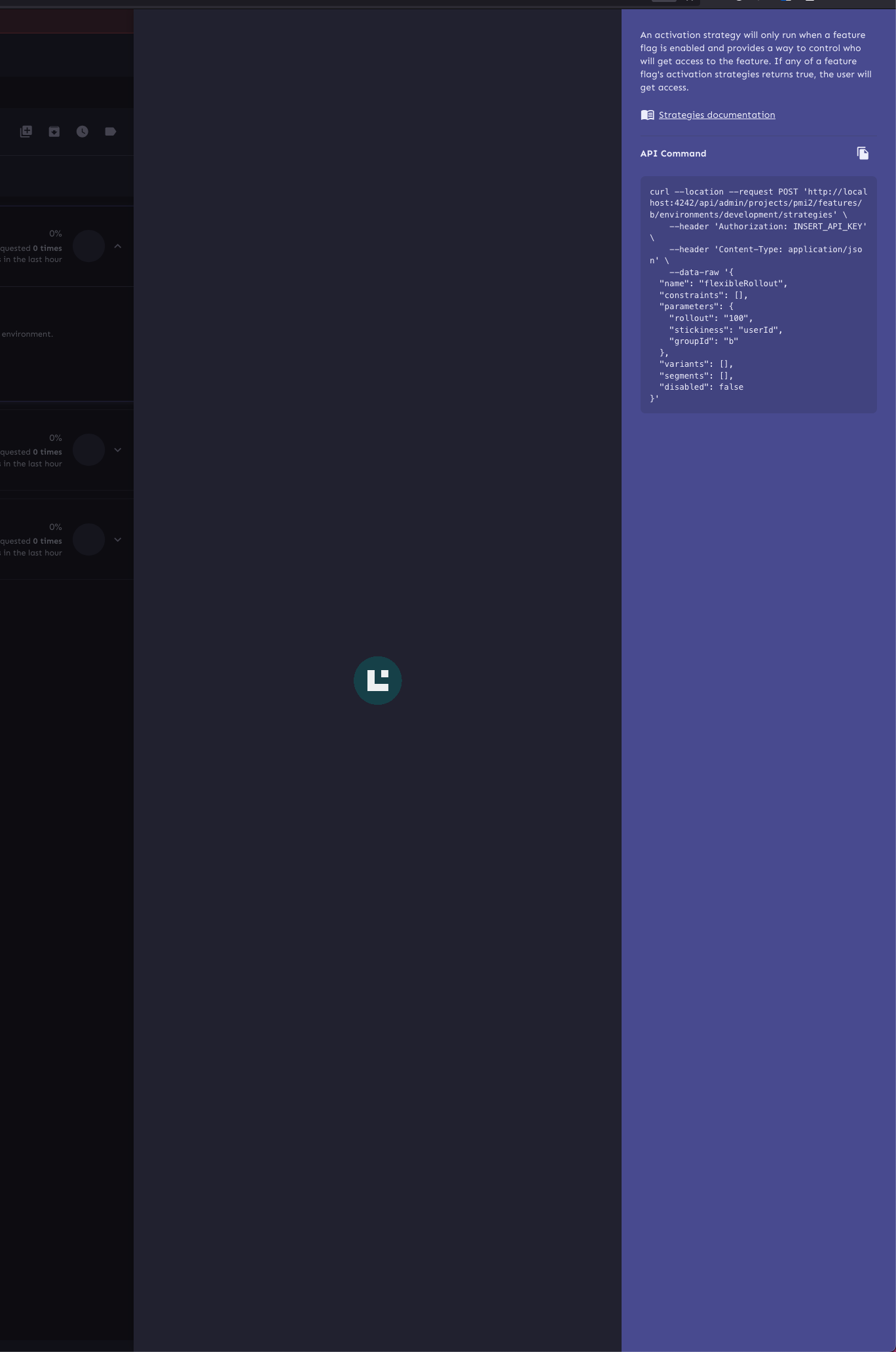 ### Advanced playground Old: 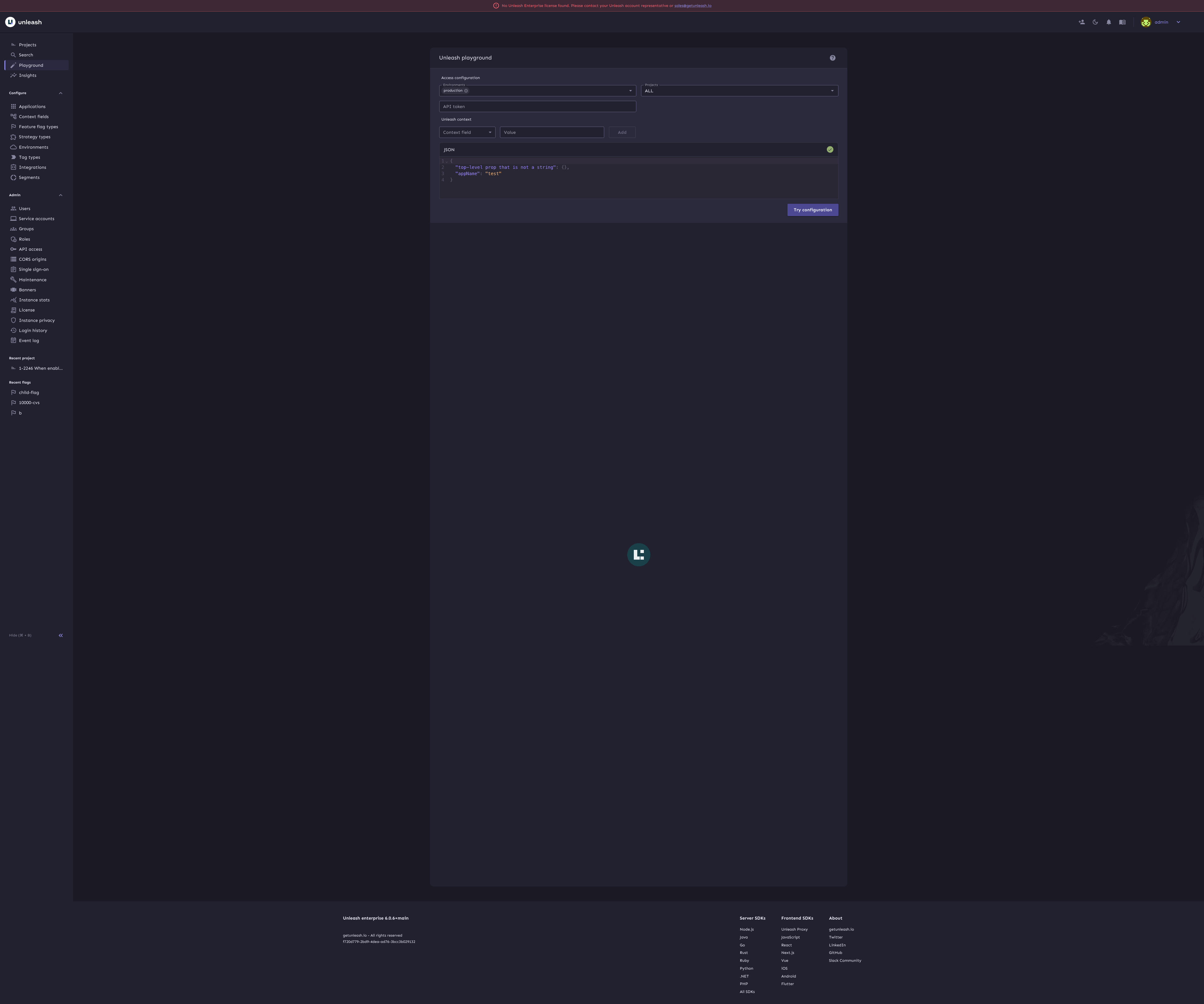 New: 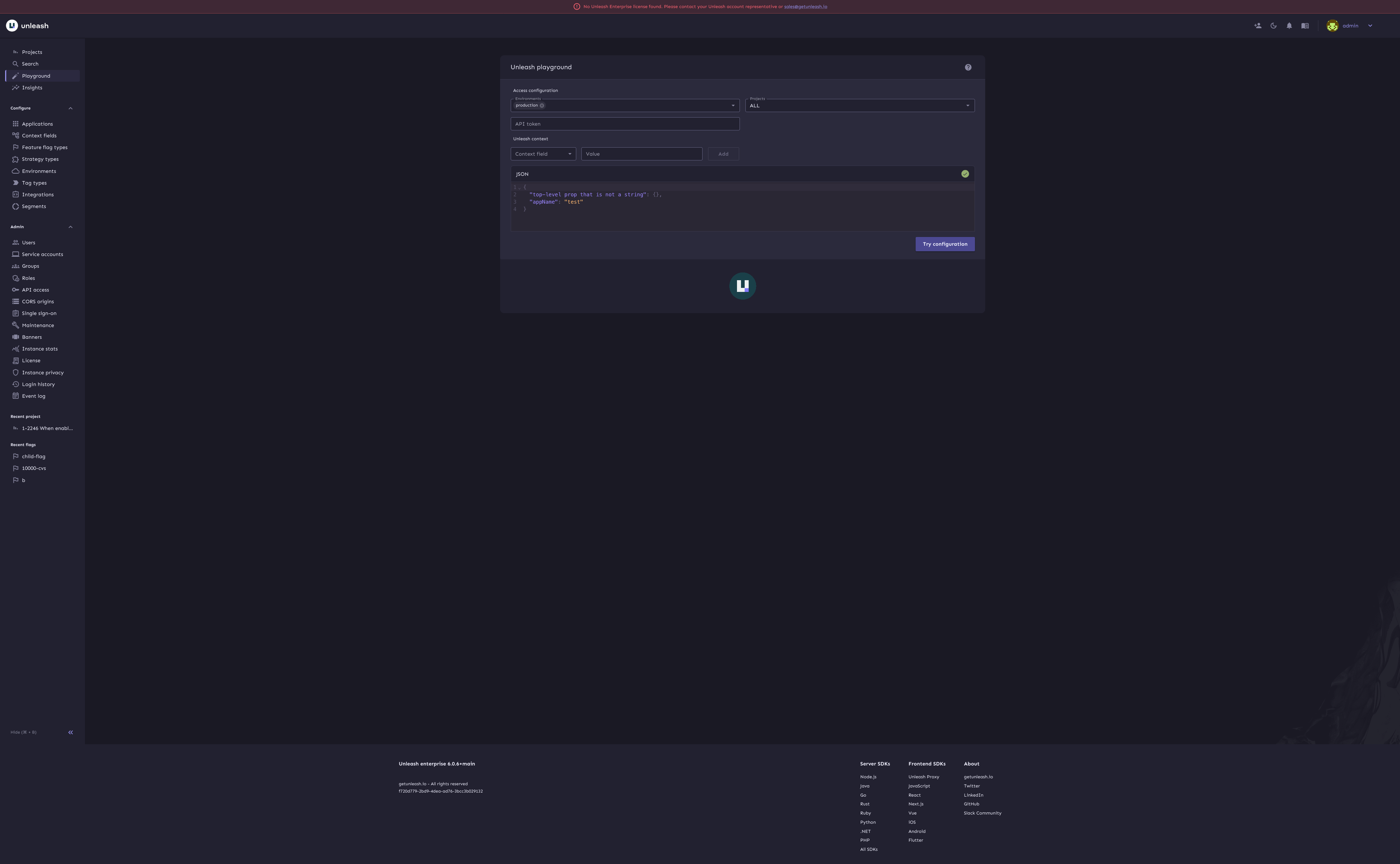 ### Loading screen / initial redirect Old: 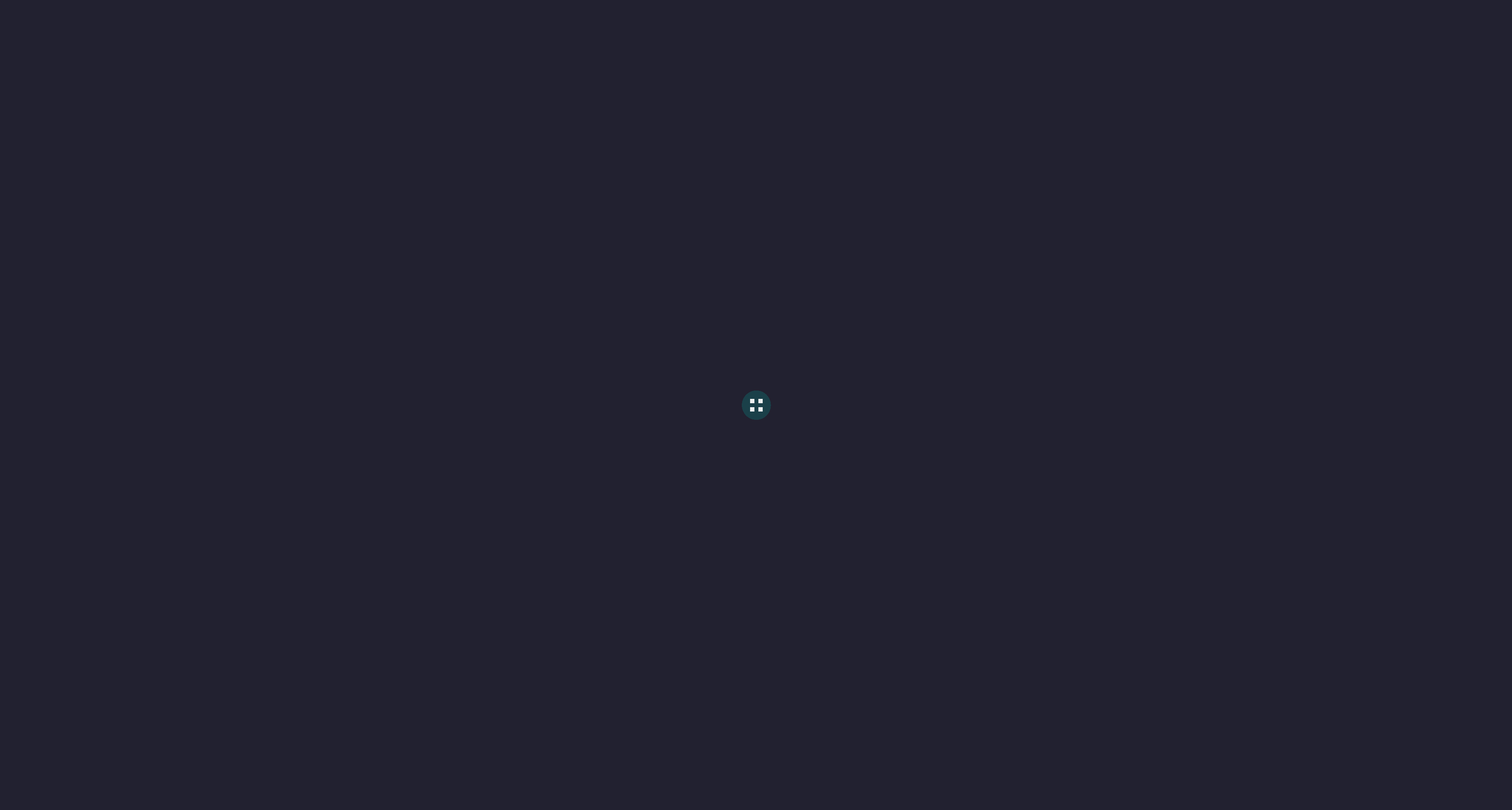 New (no new component props): 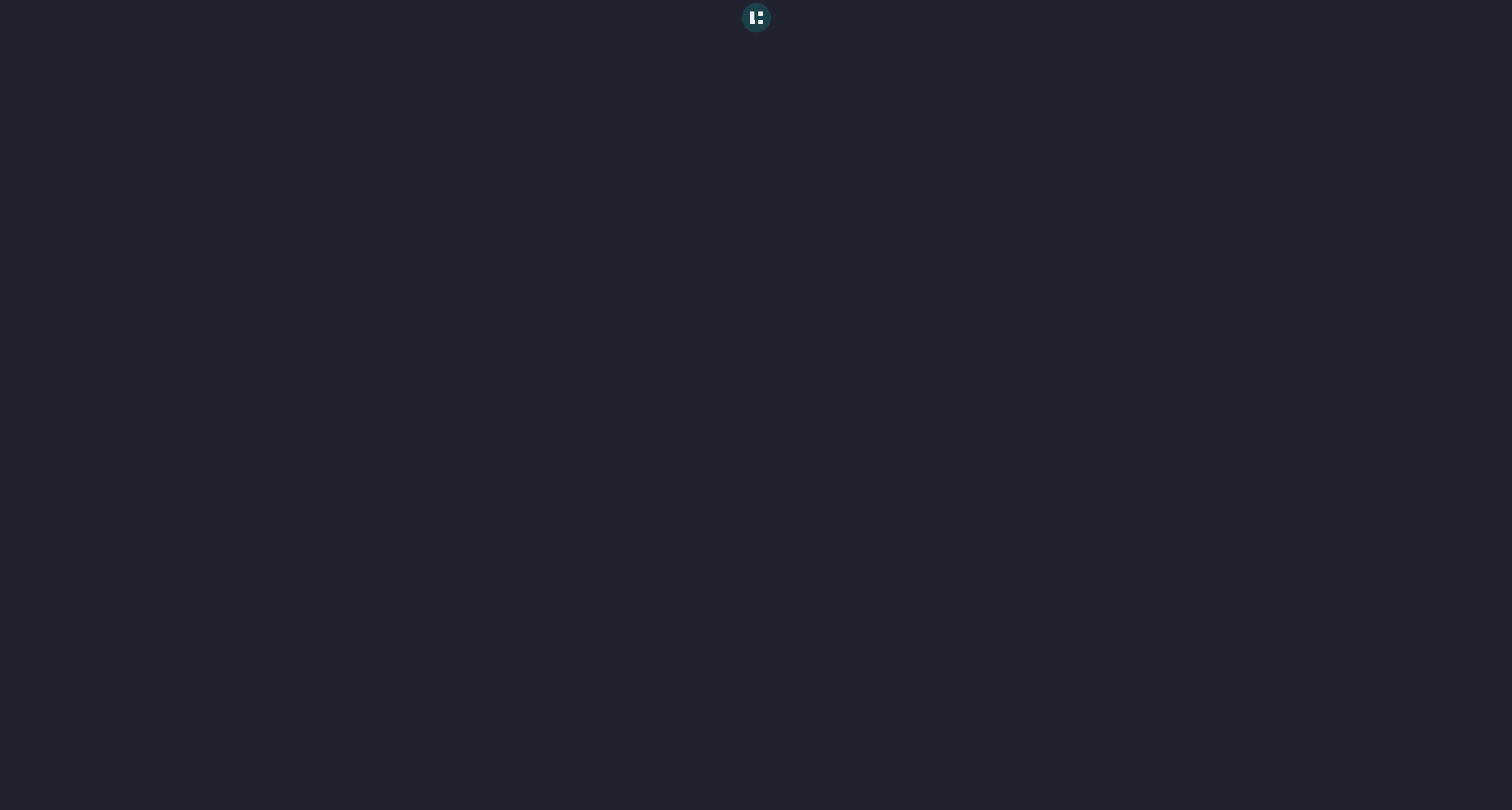 New (with new props): 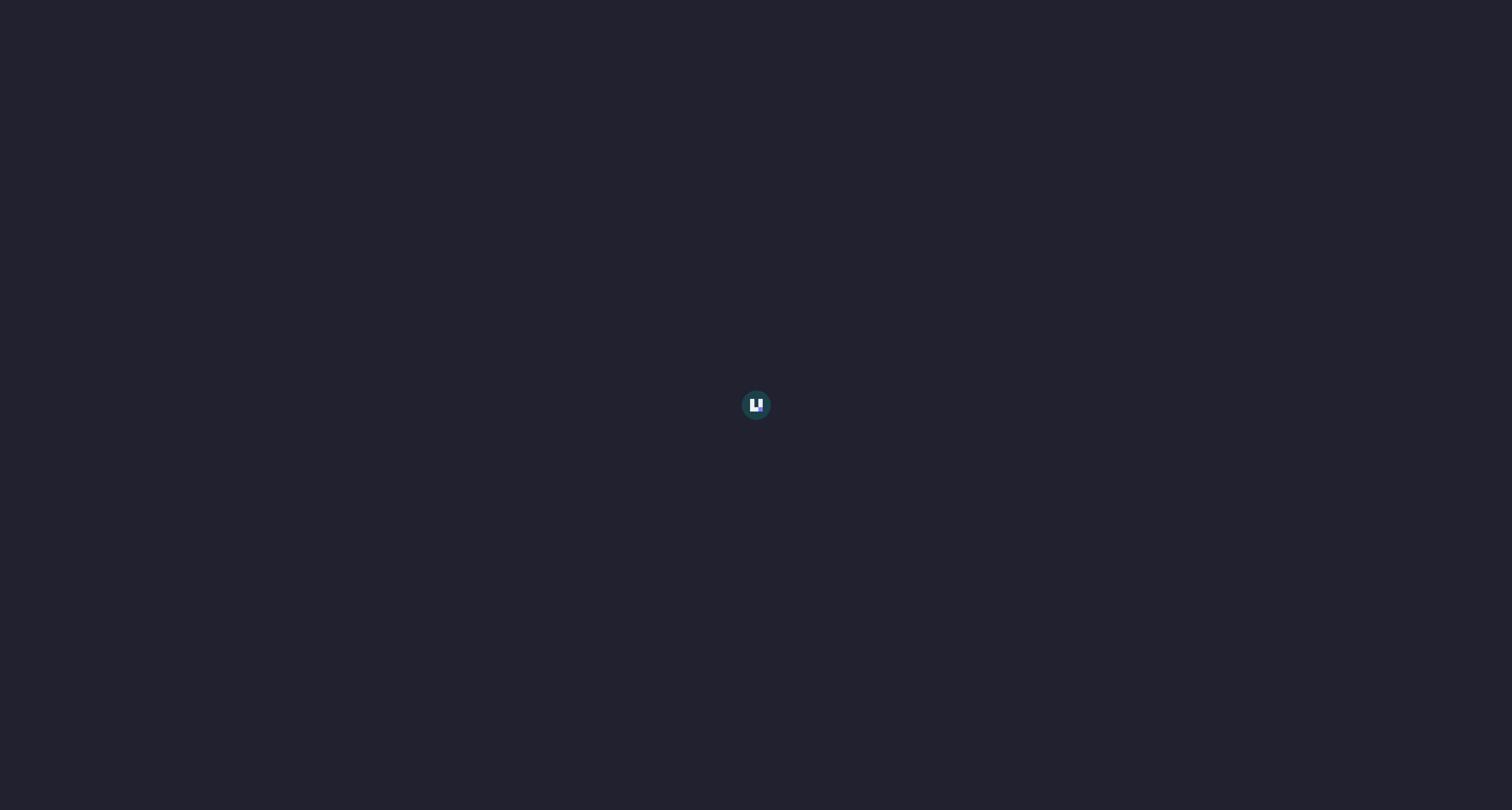
113 lines
5.0 KiB
TypeScript
113 lines
5.0 KiB
TypeScript
import { Suspense, useEffect } from 'react';
|
|
import { Route, Routes } from 'react-router-dom';
|
|
import { ConditionallyRender } from 'component/common/ConditionallyRender/ConditionallyRender';
|
|
import { FeedbackNPS } from 'component/feedback/FeedbackNPS/FeedbackNPS';
|
|
import { LayoutPicker } from 'component/layout/LayoutPicker/LayoutPicker';
|
|
import Loader from 'component/common/Loader/Loader';
|
|
import NotFound from 'component/common/NotFound/NotFound';
|
|
import { ProtectedRoute } from 'component/common/ProtectedRoute/ProtectedRoute';
|
|
import { SWRProvider } from 'component/providers/SWRProvider/SWRProvider';
|
|
import ToastRenderer from 'component/common/ToastRenderer/ToastRenderer';
|
|
import { routes } from 'component/menu/routes';
|
|
import { useAuthDetails } from 'hooks/api/getters/useAuth/useAuthDetails';
|
|
import { useAuthUser } from 'hooks/api/getters/useAuth/useAuthUser';
|
|
import { SplashPageRedirect } from 'component/splash/SplashPageRedirect/SplashPageRedirect';
|
|
import useUiConfig from 'hooks/api/getters/useUiConfig/useUiConfig';
|
|
|
|
import MaintenanceBanner from './maintenance/MaintenanceBanner';
|
|
import { styled } from '@mui/material';
|
|
import { InitialRedirect } from './InitialRedirect';
|
|
import { InternalBanners } from './banners/internalBanners/InternalBanners';
|
|
import { ExternalBanners } from './banners/externalBanners/ExternalBanners';
|
|
import { EdgeUpgradeBanner } from './banners/EdgeUpgradeBanner/EdgeUpgradeBanner';
|
|
import { LicenseBanner } from './banners/internalBanners/LicenseBanner';
|
|
import { Demo } from './demo/Demo';
|
|
|
|
const StyledContainer = styled('div')(() => ({
|
|
'& ul': {
|
|
margin: 0,
|
|
},
|
|
}));
|
|
|
|
export const App = () => {
|
|
const { authDetails } = useAuthDetails();
|
|
const { refetch: refetchUiConfig } = useUiConfig();
|
|
|
|
const { user } = useAuthUser();
|
|
const hasFetchedAuth = Boolean(authDetails || user);
|
|
|
|
const { isOss, uiConfig } = useUiConfig();
|
|
|
|
const availableRoutes = isOss()
|
|
? routes.filter((route) => !route.enterprise)
|
|
: routes;
|
|
|
|
useEffect(() => {
|
|
if (hasFetchedAuth && Boolean(user?.id)) {
|
|
refetchUiConfig();
|
|
}
|
|
}, [authDetails, user]);
|
|
|
|
return (
|
|
<SWRProvider>
|
|
<Suspense fallback={<Loader type='fullscreen' />}>
|
|
<ConditionallyRender
|
|
condition={!hasFetchedAuth}
|
|
show={<Loader type='fullscreen' />}
|
|
elseShow={
|
|
<Demo>
|
|
<>
|
|
<ConditionallyRender
|
|
condition={Boolean(
|
|
uiConfig?.maintenanceMode,
|
|
)}
|
|
show={<MaintenanceBanner />}
|
|
/>
|
|
<LicenseBanner />
|
|
<ExternalBanners />
|
|
<InternalBanners />
|
|
<EdgeUpgradeBanner />
|
|
<StyledContainer>
|
|
<ToastRenderer />
|
|
<Routes>
|
|
{availableRoutes.map((route) => (
|
|
<Route
|
|
key={route.path}
|
|
path={route.path}
|
|
element={
|
|
<LayoutPicker
|
|
isStandalone={
|
|
route.isStandalone ===
|
|
true
|
|
}
|
|
>
|
|
<ProtectedRoute
|
|
route={route}
|
|
/>
|
|
</LayoutPicker>
|
|
}
|
|
/>
|
|
))}
|
|
<Route
|
|
path='/'
|
|
element={<InitialRedirect />}
|
|
/>
|
|
<Route
|
|
path='*'
|
|
element={<NotFound />}
|
|
/>
|
|
</Routes>
|
|
|
|
<FeedbackNPS openUrl='http://feedback.unleash.run' />
|
|
|
|
<SplashPageRedirect />
|
|
</StyledContainer>
|
|
</>
|
|
</Demo>
|
|
}
|
|
/>
|
|
</Suspense>
|
|
</SWRProvider>
|
|
);
|
|
};
|